用c语言按一下要求写一篇代码1. 请以“后插法”创建一个数据元素为整数的单链表。 2. 请编写一个print函数输出单链表中的所有整数。 3. 请编写一个Max函数获取单链表中包含的最大整数值。
时间: 2024-10-16 15:06:27 浏览: 29
在C语言中,我们可以使用结构体来表示链表节点,并使用指针来处理链表操作。以下是按照你提供的要求编写的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct ListNode {
int data; // 数据域存储整数
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 后插法创建链表
ListNode* insertNode(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
return newNode;
}
// 打印链表中的所有整数
void printList(ListNode* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
// 获取链表中最大值的函数
int findMax(ListNode* head) {
if (head == NULL) {
return -1; // 如果链表为空,返回-1作为默认最大值
}
int maxVal = head->data;
ListNode* current = head->next;
while (current != NULL) {
if (maxVal < current->data) {
maxVal = current->data;
}
current = current->next;
}
return maxVal;
}
int main() {
// 初始化一个空链表
ListNode* head = NULL;
// 插入一些整数到链表
head = insertNode(&head, 5);
head = insertNode(&head, 2);
head = insertNode(&head, 8);
head = insertNode(&head, 3);
// 输出链表并打印最大值
printf("Chain list elements: ");
printList(head);
int maxInList = findMax(head);
printf("Max value in the list is: %d\n", maxInList);
return 0;
}
```
阅读全文
相关推荐
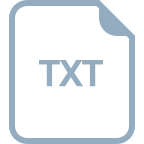
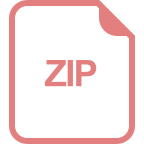











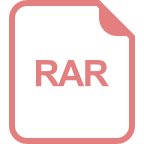
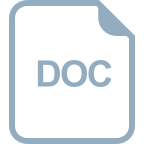


