在Python中,如何运用高斯朴素贝叶斯方法处理一个多类别(比如四类)的数据集,并展示分类结果的可视化图表?
时间: 2024-10-29 18:09:04 浏览: 19
在Python中,可以使用`sklearn`库中的`GaussianNB`(高斯朴素贝叶斯)类来处理多类别数据。首先,你需要安装`scikit-learn`库,如果尚未安装,可以使用以下命令:
```bash
pip install scikit-learn
```
下面是一个简单的步骤说明:
1. **数据预处理**:导入必要的库并加载数据。假设你的数据存储在`df` Pandas DataFrame中,其中包含特征列和目标标签列。
```python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import classification_report, confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
# 加载数据并编码类别
X = df.drop('target_column', axis=1) # 假设'target_column'是你要预测的目标列
y = df['target_column']
le = LabelEncoder()
y_encoded = le.fit_transform(y)
```
2. **拆分数据**:将数据划分为训练集和测试集。
```python
X_train, X_test, y_train, y_test = train_test_split(X, y_encoded, test_size=0.2, random_state=42)
```
3. **创建模型并训练**:
```python
gnb = GaussianNB()
gnb.fit(X_train, y_train)
```
4. **预测并评估**:
```python
y_pred = gnb.predict(X_test)
print("Classification Report:\n", classification_report(y_test, y_pred))
conf_mat = confusion_matrix(y_test, y_pred)
sns.heatmap(conf_mat, annot=True, cmap='Blues')
plt.xlabel('Predicted')
plt.ylabel('True')
plt.show()
```
在这个例子中,`classification_report`会提供每个类别的精确度、召回率和F1分数,而`confusion_matrix`则显示了实际结果与预测结果之间的对比,用于可视化模型性能。
阅读全文
相关推荐
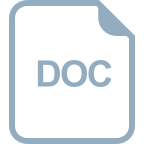
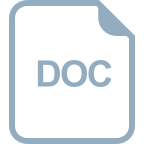
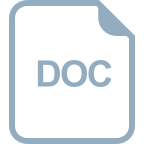
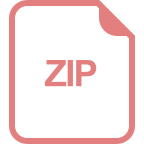
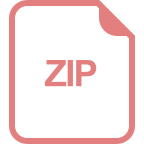
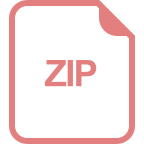
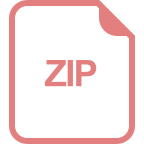
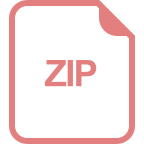
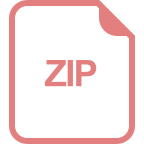
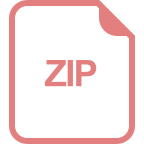
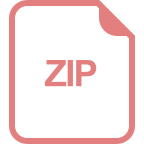
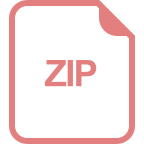
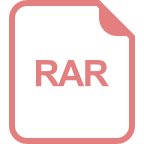
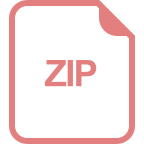
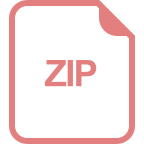



