next_permutation
时间: 2023-09-02 16:08:58 浏览: 51
`next_permutation` is a standard library function in C++ that generates the next lexicographically greater permutation of a sequence. It rearranges the elements in the range [first, last) into the next lexicographically greater permutation, where the order is determined by the comparison operator `less than` (`<`).
The function returns `true` if the next permutation exists and `false` if it does not. If `true` is returned, the function rearranges the elements in the range [first, last) to the next lexicographically greater permutation.
The `next_permutation` function takes two iterators as arguments: `first` and `last`, which define the range of elements to be rearranged. It works by finding the rightmost element in the range that is smaller than the element to its right, and then swapping it with the smallest element to its right that is greater than it. After the swap, it sorts the remaining elements in ascending order.
Here is an example usage of `next_permutation`:
```
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3};
do {
for (int i : v) {
std::cout << i << " ";
}
std::cout << std::endl;
} while (std::next_permutation(v.begin(), v.end()));
return 0;
}
```
This program generates all permutations of the sequence {1, 2, 3} and outputs them to the console. The `do-while` loop runs `next_permutation` on the vector `v` until it returns `false`, which means all permutations have been generated.
相关推荐
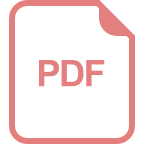
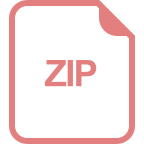
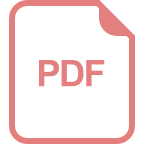










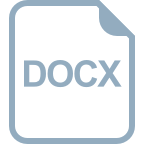
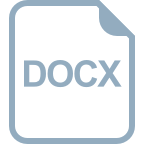
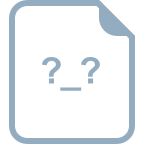
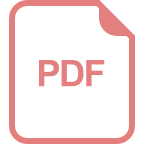