C语言统计数字字符的个数
时间: 2024-12-19 11:01:10 浏览: 9
在C语言中,统计字符串中特定字符(如数字字符 '0' 到 '9')的个数通常通过遍历字符串并逐个判断字符是否属于数字来进行。下面是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符种类的函数
int count_digits(char *str) {
int count = 0;
while (*str != '\0') { // 遍历直到遇到字符串结束符
if (isdigit(*str)) { // 使用isdigit()函数检查字符是否为数字
count++; // 如果是数字,计数器加一
}
str++; // 移动指针到下一个字符
}
return count; // 返回数字字符的总个数
}
int main() {
char input[] = "Hello123World456";
int digit_count = count_digits(input);
printf("The number of digits in the string is: %d\n", digit_count);
return 0;
}
```
在这个例子中,`count_digits` 函数接收一个字符串 `str`,它会计算并返回该字符串中数字字符的数量。`isdigit()` 函数用于检查字符是否是数字。
相关问题
c语言 统计数字字符 个数
在C语言中,统计字符串中特定字符的个数通常涉及到遍历数组或者字符串。你可以使用`for`循环配合`if`条件判断来完成这个任务。下面是一个简单的例子,假设你想统计字符串中某个字符`c`的数量:
```c
#include <stdio.h>
#include <string.h>
int count_char(char str[], char c) {
int count = 0;
for(int i = 0; str[i] != '\0'; i++) { // 遍历直到遇到字符串结束符'\0'
if(str[i] == c) { // 如果当前字符等于目标字符
count++; // 计数器加一
}
}
return count;
}
int main() {
char input[] = "Hello, World!";
char target_char = 'o';
int result = count_char(input, target_char);
printf("字符'%c'在字符串中出现了 %d 次.\n", target_char, result);
return 0;
}
```
在这个程序中,`count_char`函数接收一个字符串和一个字符作为输入,返回该字符在字符串中出现的次数。
c语言统计数字字符个数
在C语言中,统计字符串中数字字符的个数可以使用标准库函数`isdigit()`以及循环结构。以下是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含isdigit() 函数
int count_digits(char *str) {
int count = 0;
while (*str != '\0') { // 遍历直到字符串结束
if (isdigit(*str)) { // 判断当前字符是否为数字
count++; // 如果是数字,计数器加一
}
str++; // 移动到下一个字符
}
return count; // 返回数字字符的个数
}
int main() {
char input[] = "abc123def456";
int digit_count = count_digits(input);
printf("输入字符串中共有 %d 个数字字符.\n", digit_count);
return 0;
}
```
在这个程序中,`count_digits`函数遍历输入的字符串,每次检查当前字符是否是数字(`isdigit()`函数返回非零值表示是数字),如果是就增加计数器。最后,主函数打印出数字字符的数量。
阅读全文
相关推荐
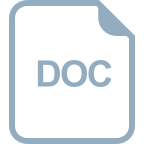
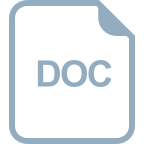
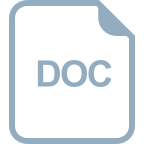













