springboot商品管理
时间: 2023-06-28 11:07:38 浏览: 63
好的,那我们就来讲一下如何使用Spring Boot来实现商品管理。
首先,我们需要创建一个新的Spring Boot项目,并添加相应的依赖。我们可以使用Spring Initializr来快速创建一个基本的Spring Boot项目。在创建项目时,我们需要选择相应的依赖,例如Web、JPA等。
接下来,我们需要定义商品的数据模型。可以使用Java类来表示商品,例如:
```java
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String description;
private BigDecimal price;
// getters and setters
}
```
上面的代码使用JPA注解来指定该类对应的数据库表名以及表中的字段。我们定义了商品的ID、名称、描述和价格属性。
接下来,我们需要创建一个Controller来处理商品的CRUD操作。Controller可以使用Spring MVC框架提供的注解来定义请求映射,例如:
```java
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductRepository productRepository;
@GetMapping
public List<Product> getAllProducts() {
return productRepository.findAll();
}
@PostMapping
public Product createProduct(@RequestBody Product product) {
return productRepository.save(product);
}
@PutMapping("/{id}")
public Product updateProduct(@PathVariable Long id, @RequestBody Product product) {
Product existingProduct = productRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("Product not found with id " + id));
existingProduct.setName(product.getName());
existingProduct.setDescription(product.getDescription());
existingProduct.setPrice(product.getPrice());
return productRepository.save(existingProduct);
}
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteProduct(@PathVariable Long id) {
Product existingProduct = productRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("Product not found with id " + id));
productRepository.delete(existingProduct);
return ResponseEntity.ok().build();
}
}
```
上面的代码使用了Spring MVC框架提供的各种注解,例如@RestController、@RequestMapping、@GetMapping、@PostMapping、@PutMapping、@DeleteMapping等。我们定义了几个请求映射来处理商品的CRUD操作,分别是获取所有商品、创建商品、更新商品、删除商品。
最后,我们需要定义一个Repository来处理商品的数据访问,例如:
```java
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
}
```
上面的代码使用了Spring Data JPA框架提供的JpaRepository接口来处理商品的数据访问。我们可以使用该接口提供的各种方法来实现商品的CRUD操作,例如findAll()、save()、findById()、delete()等。
以上就是使用Spring Boot实现商品管理的基本流程。当然,还可以添加各种其他的功能,例如商品分类、搜索、排序等。
相关推荐
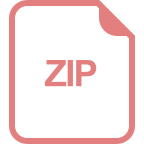
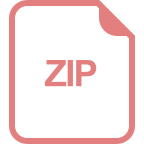
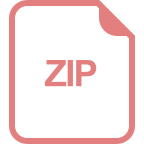
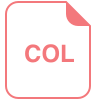












