react native NFC组件
时间: 2023-11-08 09:49:20 浏览: 187
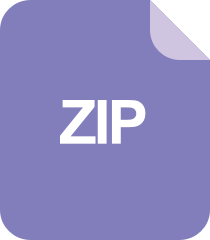
react-native-nfc-manager:用于Android和iOS的React Native NFC模块
React Native 并没有内置的 NFC 组件,但可以使用第三方库来实现 NFC 功能。以下是一些常用的 NFC 库:
1. react-native-nfc-manager:这是一个可用于 Android 和 iOS 的库,它提供了对 NFC 功能的访问权限,包括读取和写入标签、监听标签扫描事件等。
2. react-native-nfc-ios:这是一个专门为 iOS 设计的库,它允许您读取和写入 NFC 标签,并且可以与 Apple Pay 集成。
3. react-native-nfc-android:这是一个专门为 Android 设计的库,它提供了对 Android Beam 和 Host Card Emulation 的支持,允许您模拟卡片以进行测试。
这些库都可以通过 npm 安装,使用它们可以轻松地在 React Native 应用程序中实现 NFC 功能。
阅读全文
相关推荐
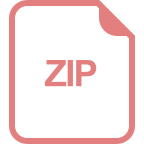
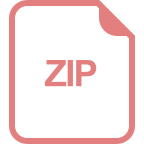




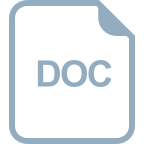

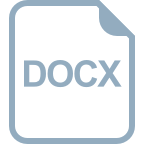
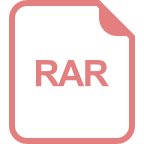
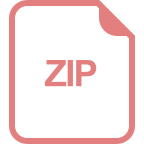
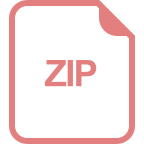
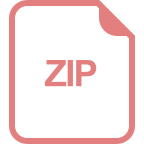
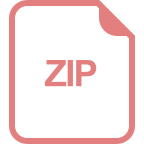