react native 实现页面组件黑白滤镜
时间: 2023-11-15 14:21:23 浏览: 170
React Native中可以使用Image组件和ImageEditor组件来实现页面组件的黑白滤镜效果。以下是实现步骤:
1. 导入Image和ImageEditor组件:
```javascript
import { Image, ImageEditor } from 'react-native';
```
2. 在组件中使用Image组件来渲染需要添加黑白滤镜的组件,并添加样式:
```javascript
<Image source={require('./assets/image.jpg')} style={styles.image} />
```
其中,require('./assets/image.jpg')指定了需要添加黑白滤镜的组件的路径,styles.image定义了Image组件的样式:
```javascript
const styles = StyleSheet.create({
image: {
width: 200,
height: 200,
},
});
```
3. 使用ImageEditor组件对组件进行黑白滤镜处理:
```javascript
ImageEditor.cropImage(uri, {
offset: { x: 0, y: 0 },
size: { width: screenWidth, height: screenHeight },
displaySize: { width: screenWidth, height: screenHeight },
resizeMode: 'contain',
}, (croppedImageURI) => {
ImageEditor.processImage(croppedImageURI, {
width: screenWidth,
height: screenHeight,
resizeMode: 'contain',
colorMatrix: [
0.33, 0.33, 0.33, 0, 0,
0.33, 0.33, 0.33, 0, 0,
0.33, 0.33, 0.33, 0, 0,
0, 0, 0, 1, 0
],
}, (processedImageURI) => {
this.setState({ processedImageURI });
}, (error) => console.error(error));
}, (error) => console.error(error));
```
其中,uri参数指定了需要添加黑白滤镜的组件的路径,colorMatrix参数指定了黑白滤镜的矩阵。处理完成后,将处理后的图片设置为组件的state,并在render方法中使用Image组件来渲染处理后的图片:
```javascript
render() {
return (
<View style={styles.container}>
<Image source={{ uri: this.state.processedImageURI }} style={styles.image} />
// 其他组件
</View>
);
}
```
注意,ImageEditor组件是异步执行的,因此需要使用回调函数来处理处理后的图片。详细使用方法可以查看React Native官方文档。
阅读全文
相关推荐
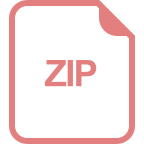
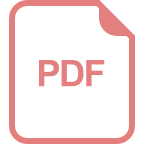
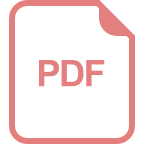















