Kotlin开发背单词app首先,使用api导入单词词库,然后编写代码将数据导入到你的app中。 在背单词页面,你需要创建一个单词列表,并在列表中显示当前单词和例句。你可以使用一个计数器来跟踪当前单词的位置,然后在点击“认识”按钮时自动切换到下一个单词。 当用户点击“不认识”按钮时,你需要显示该单词的意思,并显示一个“下一个”按钮,以便用户可以切换到下一个单词。如果用户想将单词加入生词本,添加一个“加入生词本”按钮。 在生词本页面,显示所有添加到生词本中的单词及其含义。用户应该能够删除不再需要的单词。 在已背单词列表页面,显示用户已经背过的所有单词,并提供删除选项。 最后,实现一个搜索功能,让用户能够搜索他们想要的单词。具体代码
时间: 2024-02-13 07:59:46 浏览: 27
很抱歉,我无法为您编写完整的代码。但我可以提供一些代码片段,帮助您开始:
1. 导入单词词库:
```kotlin
// 创建Retrofit实例
val retrofit = Retrofit.Builder()
.baseUrl(API_URL)
.addConverterFactory(GsonConverterFactory.create())
.build()
// 创建API接口实例
val api = retrofit.create(DictionaryAPI::class.java)
// 发起请求获取单词列表
val call = api.getWords()
call.enqueue(object : Callback<List<Word>> {
override fun onResponse(call: Call<List<Word>>, response: Response<List<Word>>) {
// 将单词列表保存到本地
val words = response.body()
saveWordsToLocal(words)
}
override fun onFailure(call: Call<List<Word>>, t: Throwable) {
// 处理错误
}
})
```
2. 创建单词列表并显示当前单词和例句:
```kotlin
// 获取单词列表
val words = getWordsFromLocal()
// 创建单词列表
val wordList = mutableListOf<Word>()
wordList.addAll(words)
// 创建计数器
var currentWordIndex = 0
// 显示当前单词和例句
val currentWord = wordList[currentWordIndex]
wordTextView.text = currentWord.word
exampleTextView.text = currentWord.example
// “认识”按钮
knowButton.setOnClickListener {
// 切换到下一个单词
currentWordIndex++
if (currentWordIndex >= wordList.size) {
currentWordIndex = 0
}
val nextWord = wordList[currentWordIndex]
wordTextView.text = nextWord.word
exampleTextView.text = nextWord.example
}
// “不认识”按钮
unknownButton.setOnClickListener {
// 显示单词意思
meaningTextView.text = currentWord.meaning
// 显示“下一个”按钮
nextButton.visibility = View.VISIBLE
}
// “下一个”按钮
nextButton.setOnClickListener {
// 隐藏“下一个”按钮
nextButton.visibility = View.GONE
// 切换到下一个单词
currentWordIndex++
if (currentWordIndex >= wordList.size) {
currentWordIndex = 0
}
val nextWord = wordList[currentWordIndex]
wordTextView.text = nextWord.word
exampleTextView.text = nextWord.example
}
```
3. 将单词加入生词本:
```kotlin
// “加入生词本”按钮
addButton.setOnClickListener {
// 将单词保存到生词本
addWordToVocabulary(currentWord)
// 显示提示信息
Toast.makeText(this, "单词已加入生词本", Toast.LENGTH_SHORT).show()
}
```
4. 显示生词本并删除单词:
```kotlin
// 获取生词本中的单词
val vocabulary = getVocabularyFromLocal()
// 创建单词列表
val wordList = mutableListOf<Word>()
wordList.addAll(vocabulary)
// 显示单词列表
val adapter = WordListAdapter(wordList)
recyclerView.adapter = adapter
// 设置删除按钮的点击事件
adapter.setOnItemClickListener(object : WordListAdapter.OnItemClickListener {
override fun onItemClick(position: Int) {
// 从生词本中删除单词
deleteWordFromVocabulary(wordList[position])
// 更新单词列表
wordList.removeAt(position)
adapter.notifyItemRemoved(position)
}
})
```
5. 显示已背单词列表并删除单词:
```kotlin
// 获取已背单词列表
val learnedWords = getLearnedWordsFromLocal()
// 创建单词列表
val wordList = mutableListOf<Word>()
wordList.addAll(learnedWords)
// 显示单词列表
val adapter = WordListAdapter(wordList)
recyclerView.adapter = adapter
// 设置删除按钮的点击事件
adapter.setOnItemClickListener(object : WordListAdapter.OnItemClickListener {
override fun onItemClick(position: Int) {
// 从已背单词列表中删除单词
deleteWordFromLearnedWords(wordList[position])
// 更新单词列表
wordList.removeAt(position)
adapter.notifyItemRemoved(position)
}
})
```
6. 实现搜索功能:
```kotlin
// 获取单词列表
val words = getWordsFromLocal()
// 创建单词列表
val wordList = mutableListOf<Word>()
wordList.addAll(words)
// 创建搜索框
searchView.setOnQueryTextListener(object : SearchView.OnQueryTextListener {
override fun onQueryTextSubmit(query: String?): Boolean {
return false
}
override fun onQueryTextChange(newText: String?): Boolean {
// 根据搜索关键字过滤单词列表
val filteredList = wordList.filter { word ->
word.word.contains(newText ?: "", true) ||
word.meaning.contains(newText ?: "", true) ||
word.example.contains(newText ?: "", true)
}
// 更新单词列表
adapter.submitList(filteredList)
return true
}
})
```
这些是一些基本的代码片段,可以帮助您开始编写背单词app。请注意,这些代码仅供参考,可能需要根据您的具体需求进行修改。
相关推荐
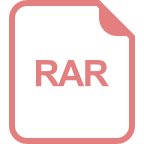
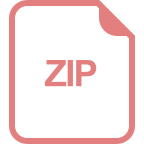














