利用卷积神经网络识别手写体python代码
时间: 2023-06-18 13:06:45 浏览: 64
识别手写体的常用方法是使用卷积神经网络(Convolutional Neural Networks,CNN),以下是一个简单的Python代码示例,可以用于训练一个CNN模型来识别手写数字:
1. 导入必要的库
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.utils import to_categorical
```
2. 加载MNIST数据集
```python
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 将数据转换为float32类型,并归一化到0~1
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
# 将标签转换为one-hot编码
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
# 将数据转换为4D张量
x_train = np.expand_dims(x_train, axis=-1)
x_test = np.expand_dims(x_test, axis=-1)
```
3. 构建CNN模型
```python
model = tf.keras.Sequential([
# 卷积层1,32个3x3的卷积核,使用relu激活函数
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
# 池化层1,2x2的池化核
tf.keras.layers.MaxPooling2D((2, 2)),
# 卷积层2,64个3x3的卷积核,使用relu激活函数
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
# 池化层2,2x2的池化核
tf.keras.layers.MaxPooling2D((2, 2)),
# 卷积层3,64个3x3的卷积核,使用relu激活函数
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
# 将张量展开成一维向量
tf.keras.layers.Flatten(),
# 全连接层1,512个神经元,使用relu激活函数
tf.keras.layers.Dense(512, activation='relu'),
# 输出层,10个神经元,使用softmax激活函数
tf.keras.layers.Dense(10, activation='softmax')
])
```
4. 编译模型
```python
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
5. 训练模型
```python
# 训练模型
model.fit(x_train, y_train, epochs=5, batch_size=64, validation_data=(x_test, y_test))
```
6. 评估模型
```python
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
以上就是一个简单的CNN模型的实现,你可以根据需要进行修改和调整。
相关推荐
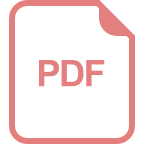


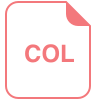
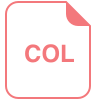
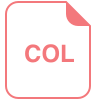









