用数据结构的方式写根据学生姓名进行字典排序,根据成绩进行由高到低排序,根据学号或姓名查找学生成绩,统计各分数段(≥90分、80-89分、70-79分、60-69分、60分以下)的学生人数及其所占比例,并打印统计的结果。手动输入学生信息
时间: 2024-03-22 11:40:37 浏览: 168
好的,这是一个比较复杂的问题,需要用到多种数据结构和算法,我将分步骤回答您的问题。
1. 学生信息的数据结构:
我们可以使用一个包含学生姓名、学号和成绩的结构体来存储每个学生的信息,如下所示:
```
struct Student {
string name;
string id;
int score;
};
```
2. 学生姓名的字典排序:
我们可以使用 STL 的 sort 函数来对学生姓名进行字典排序,代码如下所示:
```
vector<Student> students; // 存储学生信息的向量
// 对学生姓名进行字典排序
sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.name < b.name;
});
```
3. 学生成绩的由高到低排序:
我们可以使用 STL 的 sort 函数来对学生成绩进行由高到低排序,代码如下所示:
```
vector<Student> students; // 存储学生信息的向量
// 对学生成绩进行由高到低排序
sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.score > b.score;
});
```
4. 根据学号或姓名查找学生成绩:
我们可以使用 STL 的 find_if 函数来查找学号或姓名匹配的学生信息,代码如下所示:
```
vector<Student> students; // 存储学生信息的向量
// 根据学号或姓名查找学生成绩
string keyword = "xxx"; // 学号或姓名关键字
auto it = find_if(students.begin(), students.end(), [keyword](const Student& s) {
return s.id == keyword || s.name == keyword;
});
if (it != students.end()) {
cout << "学生 " << it->name << " 的成绩是 " << it->score << endl;
} else {
cout << "没有找到学号或姓名为 " << keyword << " 的学生" << endl;
}
```
5. 统计各分数段的学生人数及其所占比例:
我们可以使用 STL 的 count_if 函数和 accumulate 函数来统计各分数段的学生人数及其所占比例,代码如下所示:
```
vector<Student> students; // 存储学生信息的向量
// 统计各分数段的学生人数及其所占比例
int count90plus = count_if(students.begin(), students.end(), [](const Student& s) {
return s.score >= 90;
});
int count80to89 = count_if(students.begin(), students.end(), [](const Student& s) {
return s.score >= 80 && s.score < 90;
});
int count70to79 = count_if(students.begin(), students.end(), [](const Student& s) {
return s.score >= 70 && s.score < 80;
});
int count60to69 = count_if(students.begin(), students.end(), [](const Student& s) {
return s.score >= 60 && s.score < 70;
});
int countBelow60 = count_if(students.begin(), students.end(), [](const Student& s) {
return s.score < 60;
});
int totalCount = students.size();
double ratio90plus = (double) count90plus / totalCount * 100;
double ratio80to89 = (double) count80to89 / totalCount * 100;
double ratio70to79 = (double) count70to79 / totalCount * 100;
double ratio60to69 = (double) count60to69 / totalCount * 100;
double ratioBelow60 = (double) countBelow60 / totalCount * 100;
cout << "90分及以上的学生人数是 " << count90plus << ",占比 " << ratio90plus << "%" << endl;
cout << "80~89分的学生人数是 " << count80to89 << ",占比 " << ratio80to89 << "%" << endl;
cout << "70~79分的学生人数是 " << count70to79 << ",占比 " << ratio70to79 << "%" << endl;
cout << "60~69分的学生人数是 " << count60to69 << ",占比 " << ratio60to69 << "%" << endl;
cout << "60分以下的学生人数是 " << countBelow60 << ",占比 " << ratioBelow60 << "%" << endl;
```
6. 手动输入学生信息:
我们可以使用 cin 函数来手动输入学生信息,如下所示:
```
vector<Student> students; // 存储学生信息的向量
// 手动输入学生信息
int n; // 学生数量
cin >> n;
for (int i = 0; i < n; i++) {
Student s;
cin >> s.name >> s.id >> s.score;
students.push_back(s);
}
```
完整的代码如下所示:
阅读全文
相关推荐
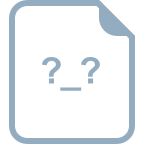










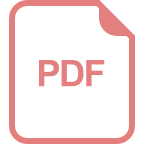
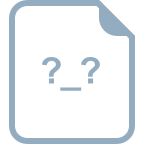
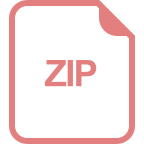
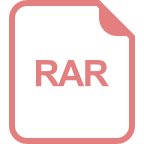
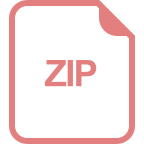