title = table.find('li',{'class':'news_title'}).text AttributeError: 'NoneType' object has no attribute 'text'
时间: 2023-06-23 21:46:54 浏览: 131
这个错误通常表示在使用find方法时,没有找到对应的HTML元素。因此,无法调用text属性。
可能的原因是:
1. HTML页面中没有包含相应的元素,或者元素的class或id属性值不正确。
2. 在使用find方法时,没有对返回值进行None的判断,导致调用None的属性而出现错误。
解决方法:
1. 确认HTML页面中是否包含了需要查找的元素,或者元素的class或id属性值是否正确。
2. 在使用find方法后,对返回值进行None的判断,例如:
```
title = table.find('li',{'class':'news_title'})
if title is not None:
title_text = title.text
else:
title_text = 'No title found'
```
相关问题
title = novel.find('h4').text AttributeError: 'NoneType' object has no attribute 'text'
这个错误通常是因为在代码中找不到指定的元素或标签,导致返回了NoneType对象。在这种情况下,我们不能对NoneType对象执行.text操作,因为它没有text属性。为了解决这个问题,我们需要检查代码中是否存在拼写错误或逻辑错误,并确保我们正在寻找正确的元素或标签。
以下是可能有用的思路和解决方案:
1. 检查代码中是否存在拼写错误或语法错误,例如括号不匹配或缺少引号等。
2. 确保我们正在寻找正确的元素或标签。可以使用浏览器的开发者工具来检查页面的HTML结构,并查找正确的元素或标签。
3. 在代码中添加一些错误处理机制,例如使用try-except语句来捕获异常并打印错误消息,以便更好地调试代码。
以下是一个可能的解决方案:
```python
novel = soup.find('div', {'class': 'novel'})
if novel is not None:
title = novel.find('h4')
if title is not None:
title_text = title.text
print(title_text)
else:
print("Title element not found")
else:
print("Novel element not found")
```
title = soup.title.string AttributeError: 'NoneType' object has no attribute 'string'
这个错误通常是因为BeautifulSoup对象没有找到符合条件的标签,导致soup.title返回None。而对None类型的对象调用string属性会出现该错误。
可能的原因有:
- HTML代码中没有<title>标签
- HTML代码中存在多个<title>标签,导致解析混乱
- 代码中使用了错误的解析器
你可以检查一下HTML代码中是否存在<title>标签,或者尝试使用其他解析器。同时,也可以使用try-except语句来处理该异常,让程序不会因为这个错误而中断。
相关推荐
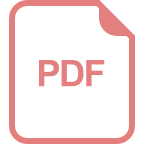
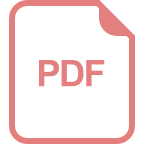
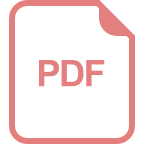












