用c语言的递归回溯算法实现轮船最大装载重量问题,不考虑物品价值,只考虑物品重量和轮船可容纳重量
时间: 2024-11-09 11:26:43 浏览: 16
在C语言中,我们可以使用递归回溯算法(Backtracking)来解决轮船最大装载问题。这个问题可以通过尝试性地选择每个容器并更新剩余承载能力的方式来实现。下面是一个简单的示例:
```c
#include <stdio.h>
// 容器容量函数,表示轮船剩余空间
int ship_capacity(int remainingWeight, int* weights, int n, int index) {
if (index == n || remainingWeight <= 0) return 0; // 所有箱子都放上或没空间了,返回剩余重量
// 如果当前箱子能放下,选择它并减去其重量
int withBox = weights[index] + ship_capacity(remainingWeight - weights[index], weights, n, index + 1);
// 如果不能放下,但下一个箱子可能可以,不选这个箱子
int withoutBox = ship_capacity(remainingWeight, weights, n, index + 1);
// 返回两者中较大的结果
return withBox > withoutBox ? withBox : withoutBox;
}
int main() {
int c = 10, n = 5;
int weights[] = {7, 2, 6, 5, 4};
printf("Maximum weight that can be loaded: %d\n", ship_capacity(c, weights, n, 0)); // 调用函数开始搜索
return 0;
}
```
在这个示例中,`ship_capacity` 函数采用递归策略遍历所有可能的装载组合。当轮船无法再装下箱子时,递归会停止。
阅读全文
相关推荐
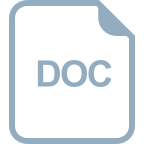
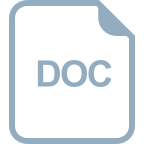
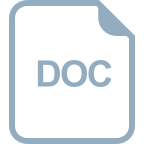
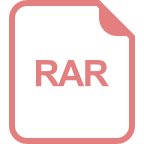
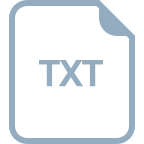
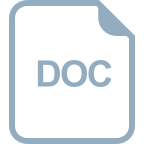
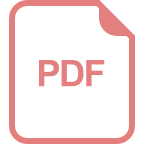
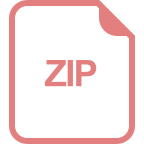
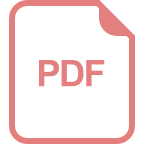
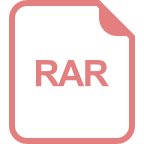
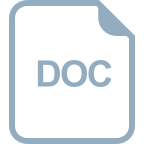
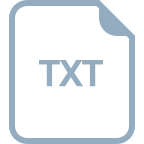
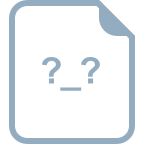
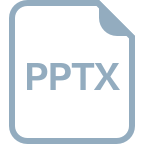
