概述python语法特点,并举例说明
时间: 2023-08-07 09:07:31 浏览: 48
Python是一种高级编程语言,其语法特点有以下几点:
1. 简洁易读:Python代码简洁易读,使用空格和缩进来表示代码块,使得代码结构清晰易懂。
例如:
```
if x < 10:
print("x is less than 10")
else:
print("x is greater than or equal to 10")
```
2. 动态强类型:Python是一种动态类型语言,变量类型不需要事先声明,可以根据赋值的对象自动推断变量类型。同时也是一种强类型语言,不同类型之间不能直接进行运算,需要进行类型转换。
例如:
```
str_var = "hello world" # str类型
int_var = 10 # int类型
float_var = 3.14 # float类型
# 普通加法运算,会报错
result = str_var + int_var + float_var # TypeError: unsupported operand type(s) for +: 'int' and 'float'
# 类型转换后正常运算
result = str_var + str(int_var) + str(float_var) # 'hello world103.14'
```
3. 面向对象:Python是面向对象的编程语言,支持类、对象、继承、封装、多态等面向对象特性。
例如:
```
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def __init__(self, name):
super().__init__(name)
def speak(self):
return "Woof!"
class Cat(Animal):
def __init__(self, name):
super().__init__(name)
def speak(self):
return "Meow!"
dog = Dog("Rufus")
cat = Cat("Whiskers")
print(dog.name + " says " + dog.speak()) # Rufus says Woof!
print(cat.name + " says " + cat.speak()) # Whiskers says Meow!
```
以上是Python语法的几个特点,它们使得Python成为一种易学易用、高效编程的语言。
相关推荐
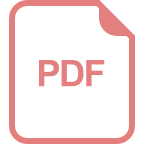
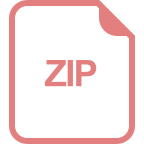














