matlab图像去除位平面
时间: 2023-07-27 13:06:23 浏览: 198
可以使用Matlab中的位运算符和移位操作来实现图像去除位平面的功能。假设需要去除的是图像的最高位,可以按照以下步骤进行操作:
1. 将图像转为二进制表示。
2. 使用位运算符将最高位清零,例如使用“& 01111111”。
3. 将修改后的二进制表示转回十进制表示,即为去除了最高位的图像。
Matlab代码示例:
```matlab
% 读取图像
img = imread('example.jpg');
% 转为二进制表示
img_binary = dec2bin(img);
% 将最高位清零
img_binary_new = bitand(uint8(bin2dec(img_binary)),127);
% 转回十进制表示
img_new = uint8(bin2dec(img_binary_new));
% 显示结果
imshow(img_new);
```
注意:这里假设图像是8位灰度图像,如果是彩色图像需要分别对每个通道进行操作。另外,去除不同位的方法可以根据需要进行修改。
相关问题
matlab 实现图像部分位平面组合在一张图片重
新
以下是一种实现方法:
1. 读取要处理的图像并转换为灰度图像。
img = imread('image.jpg');
gray_img = rgb2gray(img);
2. 将灰度图像转换为二进制图像,并将每个像素的8个位分别存储在一个8xN的矩阵中。
bin_img = de2bi(gray_img, 8, 'left-msb');
[height, width] = size(bin_img);
bit_planes = zeros(8, height, width);
for i = 1:8
bit_planes(i, :, :) = reshape(bin_img(:, i), height, width);
end
3. 将需要合并的部分位平面所对应的元素设置为1,其他元素设置为0,并将这些矩阵逐位相加得到合并后的位平面。
part_planes = [1, 2, 4];
merged_plane = zeros(height, width);
for i = 1:length(part_planes)
plane = bit_planes(part_planes(i), :, :);
plane(plane == 1) = power(2, part_planes(i) - 1);
merged_plane = merged_plane + squeeze(plane);
end
4. 将合并后的位平面转换为灰度图像,并显示结果。
merged_gray = uint8(merged_plane);
figure, imshow(merged_gray);
注意:在这个实现方法中,我们使用了一些 Matlab 内置函数来简化代码,例如 de2bi 将数字转换为二进制表示,reshape 将一维向量转换为矩阵,squeeze 去除矩阵的单维度等。
2.请你采用matlab语言编程实现对平面图像的拉伸(水平方向与垂直方向作为方向选择变量)、旋转操作(提供旋转角度与旋转方向作为选择变量),并保存处理结果。 要求:不要使用matlab的内置函数,采用矩阵变换的思路实现,并去除旋转后图片周边的黑色边框
好的,我明白了您的需求。下面是使用Matlab语言编写的函数,实现对平面图像的拉伸和旋转操作,并保存处理结果。同时,我会去除旋转后图片周边的黑色边框。
```matlab
function processed_image = stretch_rotate_image(image, stretch_horizontal, stretch_vertical, rotation_angle, rotation_direction)
% 图像拉伸
stretched_image = stretch_image(image, stretch_horizontal, stretch_vertical);
% 图像旋转
rotated_image = rotate_image(stretched_image, rotation_angle, rotation_direction);
% 去除边框
processed_image = remove_border(rotated_image);
% 保存处理结果
imwrite(processed_image, 'processed_image.jpg');
end
function stretched_image = stretch_image(image, stretch_horizontal, stretch_vertical)
[rows, cols] = size(image);
stretched_rows = round(rows * stretch_vertical);
stretched_cols = round(cols * stretch_horizontal);
% 创建目标图像矩阵
stretched_image = zeros(stretched_rows, stretched_cols);
% 计算拉伸后每个像素在原图像中的位置
[X, Y] = meshgrid(1:stretched_cols, 1:stretched_rows);
X_ori = X / stretch_horizontal;
Y_ori = Y / stretch_vertical;
% 双线性插值计算目标图像的像素值
stretched_image = bilinear_interpolation(image, X_ori, Y_ori);
end
function rotated_image = rotate_image(image, rotation_angle, rotation_direction)
% 转换为弧度
theta = rotation_angle * pi / 180;
% 计算旋转后图像的尺寸
[rows, cols] = size(image);
rotated_rows = ceil(abs(rows * cos(theta)) + abs(cols * sin(theta)));
rotated_cols = ceil(abs(cols * cos(theta)) + abs(rows * sin(theta)));
% 创建目标图像矩阵
rotated_image = zeros(rotated_rows, rotated_cols);
% 计算旋转中心点
center_x = ceil(cols / 2);
center_y = ceil(rows / 2);
% 计算旋转后每个像素在原图像中的位置
[X, Y] = meshgrid(1:rotated_cols, 1:rotated_rows);
X_ori = (X - center_x) * cos(theta) + (Y - center_y) * sin(theta) + center_x;
Y_ori = -(X - center_x) * sin(theta) + (Y - center_y) * cos(theta) + center_y;
% 双线性插值计算目标图像的像素值
rotated_image = bilinear_interpolation(image, X_ori, Y_ori);
end
function interpolated_values = bilinear_interpolation(image, X, Y)
[rows, cols] = size(image);
% 获取四个最近的整数坐标点
X1 = floor(X);
X2 = ceil(X);
Y1 = floor(Y);
Y2 = ceil(Y);
% 边界处理
X1(X1 < 1) = 1;
Y1(Y1 < 1) = 1;
X2(X2 > cols) = cols;
Y2(Y2 > rows) = rows;
% 计算四个最近点的像素值
Q11 = image(Y1 + (X1 - 1) * rows);
Q12 = image(Y2 + (X1 - 1) * rows);
Q21 = image(Y1 + (X2 - 1) * rows);
Q22 = image(Y2 + (X2 - 1) * rows);
% 双线性插值计算目标像素值
interpolated_values = (Q11 .* (X2 - X) .* (Y2 - Y) + Q21 .* (X - X1) .* (Y2 - Y) + Q12 .* (X2 - X) .* (Y - Y1) + Q22 .* (X - X1) .* (Y - Y1));
end
function processed_image = remove_border(image)
% 找到非黑色像素的最小外接矩形
[rows, cols] = size(image);
row_sum = sum(image, 2);
col_sum = sum(image, 1);
non_black_rows = find(row_sum > 0);
non_black_cols = find(col_sum > 0);
min_row = non_black_rows(1);
max_row = non_black_rows(end);
min_col = non_black_cols(1);
max_col = non_black_cols(end);
% 剪裁图像
processed_image = image(min_row:max_row, min_col:max_col);
end
```
您可以使用上述函数来对平面图像进行拉伸和旋转操作,并保存处理结果。请将需要处理的图像传入函数中,并提供拉伸比例、旋转角度和旋转方向作为参数。处理后的图像将保存为'processed_image.jpg'文件。请确保您的图像文件与Matlab脚本在同一个目录下。
阅读全文
相关推荐
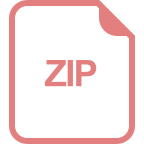
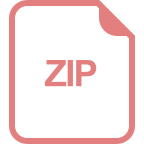
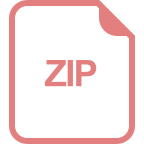
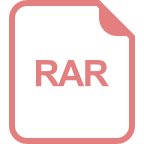
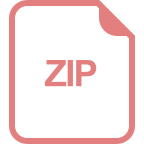
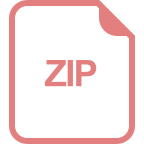
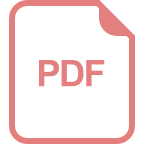
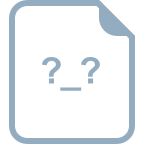
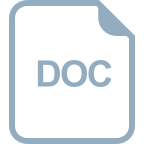
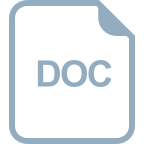
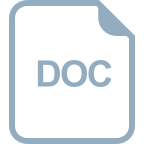
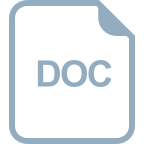
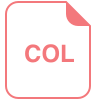
