python gnn+lstm
时间: 2023-09-19 08:01:59 浏览: 82
Python中的GNN(Graph Neural Network,图神经网络)结合LSTM(Long Short-Term Memory,长短期记忆网络)是一种强大的深度学习模型,用于处理图数据。
GNN是一类专门用于处理图数据的神经网络。它通过将节点与其邻居节点的信息进行交互来学习节点的表示。对于每个节点,GNN会将其自身特征与邻居节点的特征进行聚合,然后通过一些神经网络模块进行更新。之后,这个过程会迭代几次,直到节点的表示达到稳定状态。
LSTM则是一种特殊的循环神经网络,可以很好地处理序列数据,并具有长期依赖和短期记忆的能力。通过巧妙地设计记忆单元和遗忘门机制,LSTM可以有效地捕捉序列数据中的重要信息,并对其进行建模。
将GNN和LSTM结合可以应对一些复杂的问题,如基于图数据的序列建模、推荐系统和传感器网络等。在该结构中,GNN负责对图数据进行建模,将节点和边的信息进行编码并聚合,生成图级别的表示。然后,LSTM可以在这些图级别的表示上进行序列建模,进一步处理和学习序列之间的关系。
Python是一种功能强大的编程语言,拥有丰富的机器学习和深度学习库。可以利用Python中的GNN和LSTM库(如PyTorch、TensorFlow等),结合图数据和序列数据,实现复杂的模型训练和推断。
总结来说,Python中的GNN和LSTM可以用于处理图数据和序列数据的问题。它们的结合可以提供强大的模型能力,用于各种任务的建模和预测。
相关问题
python实现GNN+LSTM
GNN+LSTM是一种结合了图神经网络和长短时记忆网络的模型,可以用于处理图数据序列。下面是一个简单的Python实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
class GNNLSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(GNNLSTM, self).__init__()
self.hidden_dim = hidden_dim
self.gcn = GCNConv(input_dim, hidden_dim)
self.lstm = nn.LSTM(hidden_dim, hidden_dim)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x, edge_index):
x = F.relu(self.gcn(x, edge_index))
x = x.unsqueeze(0)
lstm_out, (h_n, c_n) = self.lstm(x)
out = self.fc(h_n.squeeze(0))
return out
```
这个模型的输入是一个节点特征矩阵x和一个边索引矩阵edge_index,输出是一个预测结果向量。在forward函数中,首先使用GCNConv对节点特征进行卷积操作,然后将结果输入到LSTM中进行序列建模,最后通过全连接层得到预测结果。
GNN+transformer
GNN+Transformer是一种结合了图神经网络(Graph Neural Network,简称GNN)和Transformer模型的方法。GNN是一种用于处理图数据的深度学习模型,它可以对节点和边进行特征表示学习,并通过消息传递和聚合操作来捕捉节点之间的关系。而Transformer是一种基于自注意力机制的模型,主要用于序列数据的建模,如自然语言处理任务中的机器翻译和文本生成。
将GNN和Transformer结合起来,可以在图数据上进行更加复杂的建模和推理。一种常见的方法是将GNN用于图数据的特征提取,然后将提取到的特征输入到Transformer中进行进一步的处理和预测。这种结合可以充分利用GNN对图结构的建模能力和Transformer对序列数据的建模能力,从而在图数据上实现更高效和准确的学习和推理。
相关问题:
1. GNN是什么?
2. Transformer是什么?
3. GNN+Transformer在哪些领域有应用?
4. GNN+Transformer相比于其他方法有什么优势?
相关推荐
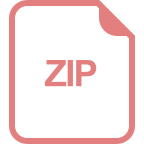
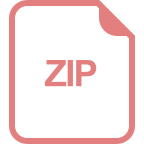
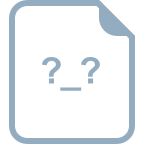











