【Python机器学习项目实战】:推荐系统构建全步骤,实战演练
发布时间: 2024-08-31 08:03:39 阅读量: 18 订阅数: 35 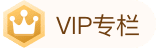
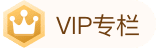
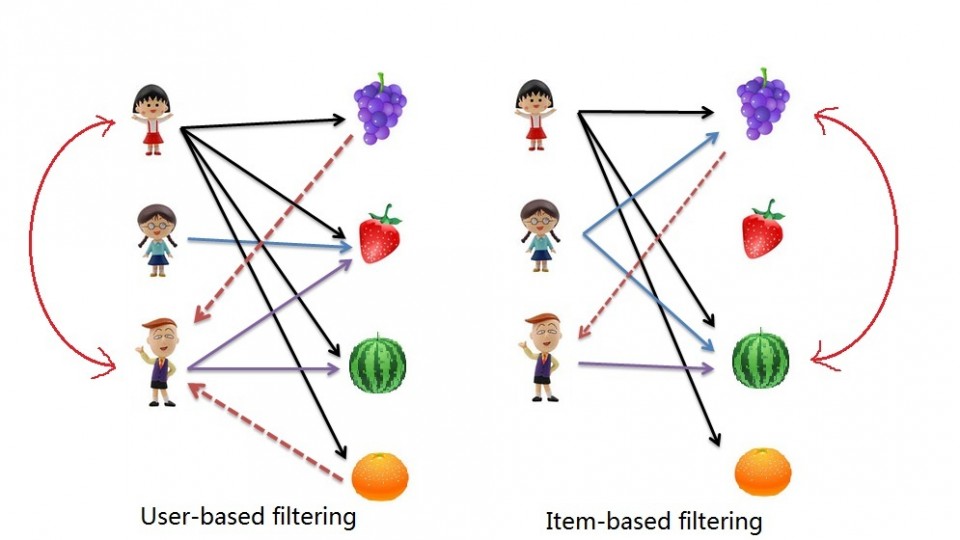
# 1. 推荐系统的概念与重要性
推荐系统作为信息技术领域的一个重要分支,它的核心目标是基于用户的行为、偏好、特征以及其他相关信息,提供个性化的信息或物品推荐。它在我们的日常生活中无处不在,例如电商平台的商品推荐、音乐流媒体的歌曲推荐、视频平台的内容推荐等。随着大数据和人工智能技术的发展,推荐系统已经从最初的基础推荐演变为具有高度智能化和个性化的现代推荐系统。
推荐系统的重要性体现在它能够极大地提升用户体验,增加用户粘性,同时对于商家和内容提供者来说,它可以帮助他们更高效地推广产品或内容,从而提高收益。例如,个性化推荐能够帮助用户快速找到他们感兴趣的商品或内容,减少搜索成本,增加购买概率。
在信息过载的时代背景下,推荐系统扮演了“信息过滤器”的角色,帮助用户从海量信息中筛选出最有价值、最相关的内容。因此,它不仅仅是提升用户体验的技术手段,更是商业决策支持系统的重要组成部分,对商家和用户都具有深远的影响。接下来的章节将深入探讨如何利用Python进行机器学习,以及如何构建高效的推荐系统。
# 2. Python机器学习基础知识
在当今的数据驱动时代,Python已成为数据分析、机器学习和人工智能的首选语言之一。Python的简洁语法、丰富的库支持以及强大的社区使得它在处理机器学习任务时表现出色。本章旨在为读者提供Python机器学习的坚实基础,内容涵盖Python编程基础、机器学习理论简介和数据预处理技术。通过深入学习本章,读者将能够理解并掌握在构建推荐系统之前所需的基本技能。
## 2.1 Python编程基础
Python编程基础是学习机器学习的前提。本小节将从数据结构和函数模块的使用两大方面,介绍Python的核心编程概念。
### 2.1.1 Python数据结构
Python提供了多种数据结构,如列表(list)、元组(tuple)、字典(dict)和集合(set),它们是构建复杂应用的基石。本段将详细介绍列表和字典的基本操作及其在机器学习中的应用。
列表是Python中最基本的数据结构之一,它是一个有序的集合,可以存储任意类型的数据。
```python
# 列表示例
fruits = ['apple', 'banana', 'cherry']
print(fruits[0]) # 访问第一个元素
# 列表切片
print(fruits[1:3]) # 访问第二个到第三个元素
```
字典是一种映射类型,它存储键值对,其中的键是唯一的。
```python
# 字典示例
person = {
'name': 'Alice',
'age': 25,
'city': 'New York'
}
# 访问字典中的值
print(person['name']) # 输出: Alice
```
### 2.1.2 函数和模块的使用
函数是组织代码的重要方式,而模块则允许我们将代码组织成更小的文件,方便复用和维护。在机器学习中,常常需要编写自定义函数来处理数据,同时导入外部模块来使用它们提供的高级功能。
```python
# 函数示例
def greet(name):
return f'Hello, {name}!'
print(greet('Alice')) # 输出: Hello, Alice!
# 模块导入示例
import math
print(math.sqrt(16)) # 输出: 4.0
```
## 2.2 机器学习理论简介
机器学习理论为推荐系统的设计提供了重要的理论基础。本小节将探讨机器学习的主要类型和一些常用的算法。
### 2.2.1 机器学习的主要类型
机器学习可以大致分为监督学习、无监督学习、半监督学习和强化学习等类型。每种类型都有其特定的应用场景。
- 监督学习:模型通过输入-输出对进行训练,预测给定输入的输出。
- 无监督学习:模型没有标签数据,而是试图找到数据内部的结构。
- 半监督学习:结合了监督学习和无监督学习的方法。
- 强化学习:模型通过与环境的交互来学习策略,以最大化某种奖励信号。
### 2.2.2 常见的机器学习算法
机器学习算法多种多样,这里仅列举几种在推荐系统中常用的算法:
- 线性回归:用于预测连续值。
- 决策树:用于分类和回归任务,通过构建决策规则来预测。
- 随机森林:通过构建多个决策树来进行预测,并对结果进行投票或平均。
- 支持向量机(SVM):一种强大的分类器,特别适用于小数据集的情况。
- k-最近邻(k-NN):一种基本的分类与回归方法,根据最近的k个邻居的特性来进行预测。
## 2.3 数据预处理技术
数据预处理是机器学习流程中不可或缺的一环,它涉及数据清洗、标准化和归一化等步骤,目的是将原始数据转化为适合模型处理的格式。
### 2.3.1 数据清洗的方法
数据清洗是识别和纠正数据中错误、不一致的过程。常见方法包括:
- 缺失值处理:通过删除、填充或插值等方法处理缺失数据。
- 噪声数据处理:使用平滑、离散化等方法减少数据的噪声。
- 异常值检测:通过统计学方法识别和处理异常值,如箱形图、Z分数等。
```python
import pandas as pd
# 示例数据
data = pd.DataFrame({
'A': [1, 2, np.nan, 4, 5],
'B': [5, np.nan, np.nan, 8, 10],
'C': [10, 20, 30, 40, 50]
})
# 缺失值处理:删除含有缺失值的行
cleaned_data = data.dropna()
# 填充缺失值为某列的平均值
data_filled = data.fillna(data.mean())
```
### 2.3.2 数据标准化和归一化
数据标准化和归一化是将数据按比例缩放,使之落入一个小的特定区间。标准化通常将数据的均值变为0,标准差变为1;归一化则通常将数据缩放到[0,1]区间。
```python
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# 数据标准化示例
scaler = StandardScaler()
data_normalized = scaler.fit_transform(data)
# 数据归一化示例
scaler = MinMaxScaler()
data_scaled = scaler.fit_transform(data)
```
以上便是Python机器学习基础知识的深入解析,理解这些概念对于构建推荐系统至关重要。接下来,在下一章节中,我们将深入了解推荐系统的各种技术,并探索如何将这些技术应用于实际项目中。
# 3. 推荐系统技术详解
## 3.1 协同过滤技术
### 3.1.1 用户基协同过滤
用户基协同过滤是推荐系统中广泛使用的一种技术,其核心思想是利用用户间的相似性来进行推荐。通过分析目标用户与其他用户在历史行为上的相似程度,从而预测目标用户对特定商品的喜好程度,并做出推荐。
用户基协同过滤的实现可以分为以下步骤:
1. **用户相似度计算**:通常使用诸如余弦相似度、皮尔逊相关系数、杰卡德相似系数等来衡量用户间的相似度。
2. **寻找最近邻用户**:选择与目标用户相似度较高的其他用户,这些用户被称为最近邻用户。
3. **生成推荐**:根据最近邻用户的喜好,为目标用户推荐相似的商品或内容。
下面是一个使用Python实现用户基协同过滤的代码示例:
```python
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# 假设 ratings 是一个用户-项目评分矩阵
ratings = np.array([
[5, 3, 0, 1],
[4, 0, 0, 1],
[1, 1, 0, 5],
[1, 0, 0, 4],
[0, 1, 5, 4],
])
# 计算用户间的相似度矩阵
user_similarity = cosine_similarity(ratings)
# 假设我们已经知道了目标用户的索引为3
target_user_index = 3
target_user_similarity = user_similarity[target_user_index]
# 对相似度进行排序,并选择相似度最高的用户
sorted_users = np.argsort(-target_user_similarity)
nearest_neighbors = sorted_users[1:6] # 选取前5个最近邻用户
# 生成推荐列表
recommendations = []
for neighbor in nearest_neighbors:
user_ratings = ratings[neighbor]
# 找到该用户评分而目标用户未评分的商品
unrated_items = np.where(user_ratings > 0)[0]
for item in unrated_items:
if ratings[target_user_index][item] == 0:
recommendations.append(item)
print("Recommended items for the target user:", sorted(recommendations))
```
在上述代码中,我们首先计算了用户间的相似度,并排序以找到最近邻用户。然后,我们推荐了那些最近邻用户评分而目标用户未评分的商品给目标用户。
### 3.1.2 物品基协同过滤
物品基协同过滤是另一种流行的推荐系统技术,与用户基协同过滤相反,物品基方法关注的是相似物品之间的推荐。
物品基协同过滤的一般步骤包括:
1. **物品相似度计算**:计算各物品间的相似度。
2. **找到目标物品的相似物品集合**:对目标物品而言,选择那些与之最相似的物品。
3. **推荐生成**:根据目标用户的已评分物品,推荐那些相似物品集合中的物品。
下面的代码演示了如何实现物品基协同过滤:
```python
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# 假设 ratings 是一个用户-项目评分矩阵
ratings = np.array([
[5, 3, 0, 1],
[4, 0, 0, 1],
[1, 1, 0, 5],
[1, 0, 0, 4],
[0, 1, 5, 4],
])
# 计算物品间的相似度矩阵
item_similarity = cosine_similarity(ratings.T)
# 假设我们已经知道了目标物品的索引为1
target_item_index = 1
target_item_similarity = item_similarity[target_item_index]
# 对相似度进行排序,并选择相似度最高的物品
sorted_items = np.argsort(-target_item_similarity)
nearest_neighbors = sorted_items[1:6] # 选取前5个最相似物品
# 推荐生成:找到已评分该物品的用户,并推荐最相似的物品
recommended_users = np.where(ratings[:, target_item_inde
```
0
0
相关推荐
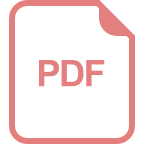
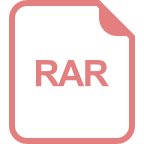






