【金融领域的Python强化学习应用】:案例研究与实战演练
发布时间: 2024-08-31 18:55:02 阅读量: 124 订阅数: 48 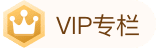
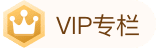
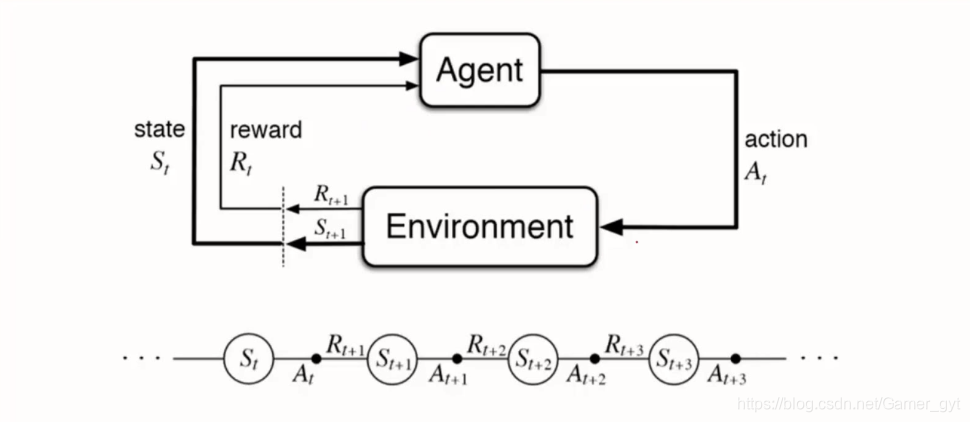
# 1. 金融领域中的Python应用概述
金融领域已经进入了数据驱动的时代,Python由于其简洁的语法、强大的数据处理能力和丰富的科学计算库,成为金融领域编程的首选。本章将浅析Python在金融领域中的应用,为后续章节对强化学习在金融领域的深入探讨做铺垫。
## 1.1 Python在金融领域的应用广泛性
Python在金融领域的应用非常广泛,从数据分析、风险管理到量化交易策略的开发,Python几乎在每个环节都扮演了重要角色。其强大的数据处理和可视化能力,使得金融分析师可以迅速从大量数据中提取有价值的见解。
## 1.2 金融工程中的Python工具
Python拥有诸如NumPy、Pandas、SciPy等强大的数学和统计库,这些工具在金融工程中的应用可以涵盖时间序列分析、期权定价模型、风险评估等。同时,它还支持机器学习、深度学习的库,如scikit-learn、TensorFlow和PyTorch等,为复杂金融问题的求解提供了新的可能性。
## 1.3 Python与金融科技创新
在金融科技(FinTech)的创新中,Python不仅促进了新兴技术的发展,如区块链、加密货币等,也推动了传统金融服务的升级。Python的易用性和灵活性使其成为金融分析师、工程师和数据科学家进行创新研究的利器。
通过本章的概述,我们可以了解到Python在金融领域的广泛应用,并为其在强化学习中的应用奠定基础。接下来的章节将更深入地探讨强化学习的理论基础以及Python如何在此领域中发挥作用。
# 2. 强化学习基础理论
在金融领域,强化学习已经成为一种有效的方法来开发和优化决策系统,特别是在动态和不确定的市场环境中。强化学习结合了机器学习和动态规划的优点,通过与环境的互动来学习最优决策策略。为了深入理解强化学习在金融中的应用,我们首先需要掌握其基础理论。
## 2.1 强化学习的数学原理
### 2.1.1 马尔可夫决策过程(MDP)
马尔可夫决策过程(Markov Decision Process,MDP)是强化学习中最基本的数学模型之一。它描述了一个决策者(或智能体)在随机环境中做出决策的框架。MDP包括以下基本要素:
- 状态空间(S):智能体可以存在于的所有状态的集合。
- 行动空间(A):智能体在每个状态下可以执行的所有可能动作的集合。
- 转移概率(P):在给定当前状态和采取某个动作的情况下,智能体转移到下一个状态的概率。
- 奖励函数(R):表示智能体在采取某动作并转移到下一状态时获得的即时奖励。
- 折扣因子(γ):未来的奖励相对于即时奖励的价值衰减程度。
在MDP中,智能体的目标是找到一种策略π,使得在该策略下长期累积的折扣奖励最大化。
#### 代码块解析
```python
import numpy as np
import matplotlib.pyplot as plt
# 假设有一个简单的MDP模型
class MDP:
def __init__(self, states, actions, transition_probabilities, rewards, gamma):
self.states = states
self.actions = actions
self.transition_probabilities = transition_probabilities
self.rewards = rewards
self.gamma = gamma
def get_next_state_reward(self, current_state, action):
# 选择动作后转移到下一个状态并获得奖励
probabilities = self.transition_probabilities[current_state][action]
next_state = np.random.choice(self.states, p=probabilities)
reward = self.rewards[current_state][action][next_state]
return next_state, reward
# 示例状态、动作、转移概率、奖励和折扣因子
states = [0, 1]
actions = ['left', 'right']
transition_probabilities = {0: {'left': [0.7, 0.3], 'right': [0.4, 0.6]},
1: {'left': [0.9, 0.1], 'right': [0.5, 0.5]}}
rewards = {0: {'left': {0: 1, 1: 0}, 'right': {0: 0, 1: 1}},
1: {'left': {0: 0, 1: 1}, 'right': {0: 1, 1: 0}}}
gamma = 0.9
# 创建MDP实例
mdp = MDP(states, actions, transition_probabilities, rewards, gamma)
# 演示MDP的转移过程
current_state = 0
action = 'right'
for _ in range(5):
next_state, reward = mdp.get_next_state_reward(current_state, action)
print(f"从状态 {current_state} 采取动作 {action},转移到状态 {next_state} 并获得奖励 {reward}")
current_state = next_state
```
上述代码块定义了一个简单的MDP类,并展示了如何使用它来模拟状态转移和奖励过程。
### 2.1.2 奖励函数与价值函数
在MDP中,奖励函数是根据当前状态和执行的动作来确定的,它反映了即时的环境反馈。价值函数则是一个更加全局的概念,它描述了在某个策略下,从当前状态开始的预期长期奖励。
- **奖励函数(R)**:R(s, a, s') 表示从状态 s 采取动作 a 并转移到新状态 s' 时获得的即时奖励。
- **价值函数(V)**:V(s) 表示从状态 s 开始,遵循策略 π 的预期长期累积奖励。
- **动作价值函数(Q)**:Q(s, a) 表示从状态 s 采取动作 a,然后遵循策略 π 的预期长期累积奖励。
价值函数可以通过贝尔曼方程进行递归计算,这是强化学习中的核心概念之一。
#### 代码块解析
```python
# 给定一个MDP模型和一个策略,计算价值函数
def compute_value_function(mdp, policy):
states = mdp.states
values = {state: 0 for state in states} # 初始化状态价值
for _ in range(100): # 迭代直到收敛
new_values = {}
for state in states:
action = policy[state]
transition_probs = mdp.transition_probabilities[state][action]
reward = mdp.rewards[state][action]
new_value = sum([p * (reward[s] + mdp.gamma * values[s]) for s, p in enumerate(transition_probs)])
new_values[state] = new_value
values = new_values
return values
# 定义一个简单的策略
policy = {0: 'left', 1: 'right'}
# 计算策略的价值函数
values = compute_value_function(mdp, policy)
print(values)
```
这段代码通过贝尔曼方程计算了一个给定策略的价值函数。它是一个迭代过程,直到价值函数收敛。
## 2.2 强化学习算法概览
### 2.2.1 Q学习与SARSA算法
Q学习和SARSA都是模型无关的强化学习算法,它们不依赖于环境的转移概率模型。这两个算法都试图直接学习一个动作价值函数Q(s, a),然后使用这个函数来指导行为。
- **Q学习**是一个异策略算法,它在计算Q值时使用贪婪策略,但在行为选择时可能使用ε-贪婪策略。
- **SARSA**是一个同策略算法,它在更新Q值时使用相同的策略,这使得它能够更稳定地收敛。
在实际应用中,选择哪一个算法取决于具体问题的性质。
#### 代码块解析
```python
# 使用Q学习算法
def q_learning(mdp, actions, learning_rate=0.1, discount_factor=0.9, episodes=1000, epsilon=0.1):
Q = {s: {a: 0 for a in actions} for s in mdp.states}
for episode in range(episodes):
state = np.random.choice(mdp.states)
done = False
while not done:
action = np.random.choice(actions) if np.random.random() < epsilon else max(Q[state], key=Q[state].get)
next_state, reward = mdp.get_next_state_reward(state, action)
Q[state][action] += learning_rate * (reward + discount_factor * max(Q[next_state].values()) - Q[state][action])
state = next_state
if state == -1: # 假设-1为终止状态
done = True
return Q
# 计算Q学习的价值函数
q_values = q_learning(mdp, actions)
print(q_values)
```
此代码段展示了Q学习算法的基本逻辑,其中包含探索(epsilon)和利用(贪婪策略)。
### 2.2.2 策略梯度方法
策略梯度方法是一类在动作空间是连续或是非有限集合时特别有用的算法。这类方法直接优化策略函数,即给定一个状态时选择动作的概率分布。
- **策略梯度方法**的核心思想是通过梯度上升来最大化期望回报。
- 这种方法通常涉及大量的采样,并通过模拟或与环境的交互来估计回报梯度。
常见的策略梯度方法包括REINFORCE算法及其变种。
#### 代码块解析
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 定义一个简单的策略网络
class PolicyNetwork(nn.Module):
def __init__(self, state_size, action_size):
super(PolicyNetwork, self).__init__()
self.fc1 = nn.Linear(state_size, 128)
self.fc2 = nn.Linear(128, action_size)
def forward(self, state):
x = torch.relu(self.fc1(state))
x = torch.softmax(self.fc2(x), dim=-1)
return x
# 模拟REINFORCE算法
def reinforce(mdp, policy_network, learning_rate=0.01, episodes=1000):
optimizer = optim.Adam(policy_network.parameters(), lr=learning_rate)
for episode in range(episodes):
state = np.random.choice(mdp.states)
log_probs = []
rewards = []
done = False
while not done:
state_tensor = torch.tensor([state]).float()
probs = policy_network(state_tensor)
action = torch.multinomial(probs, 1).item()
next_state, reward = mdp.get_next_state_reward(state, actions[action])
log_probs.append(torch.log(probs[action]))
rewards.append(reward)
state = next_state
if state == -1:
done = True
total_reward = sum(rewards)
R = 0
policy_loss = []
for r in reversed(rewards):
R = r + discount_factor * R
policy_loss.append(-log_probs.pop(0) * R)
policy_loss.reverse()
policy_loss = torch.cat(policy_loss).sum()
optimizer.zero_grad()
policy_loss.backward()
optimizer.step()
return policy_network
# 创建网络实例并运行REINFORCE算法
policy_network = PolicyNetwork(len(mdp.states), len(actions))
trained_policy = reinforce(mdp, policy_network)
```
这个代码块展示了REINFORCE算法的基本实现,使用了神经网络来参数化策略函数,并通过反向传播来更新策略网络的权重。
### 2.2.3 深度Q网络(DQN)
深度Q网络(Deep Q-Network,DQN)是结合了深度学习和Q学习的突破性方法,它使用深度神经网络来逼近Q值函数。DQN解决了传统Q学习在高维状态空间中的困难,并且能够处理更加复杂的问题。
- **DQN的关键创新包括**:使用经验回放(experience replay)和目标网络(target network)来稳定训练。
- 经验回放是指将经验(状态、动作、奖励等)存储在回放缓冲区中,然后在学习过程中随机抽取一批经验进行更新,从而打破时间相关性。
- 目标网络是另一个用于计算目标Q值的神经网络,它与行为网络同构,但参数更新更缓慢。
#### 代码块解析
```python
import random
from collections import deque
# 经验回放池
experience_replay = deque(maxlen=10000)
# 存储经验(s, a, r, s')
def store_experience(state, action, reward, next_state):
experience_replay.append((state, action, reward, next_state))
# DQN算法的更新步骤
def dqn_update(batch_size):
minibatch = random.sample(experience_replay, batch_size)
for state, action, reward, next_state in minibatch:
current_q_values = q_net(torch.tensor(state).float())
next_q_values = target_q_net(torch.tensor(next_state).float())
target = reward + discount_factor * torch.max(next_q_va
```
0
0
相关推荐
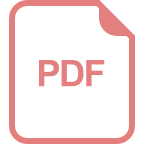
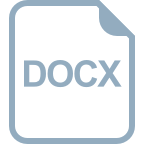
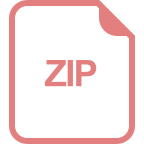
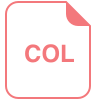
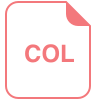
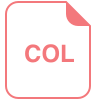
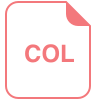
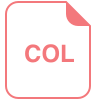
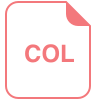