opencv-python通过dlib人脸关键点实现嘴巴和眼睛分割
时间: 2024-02-16 07:03:36 浏览: 257
是的,你可以使用OpenCV-Python和dlib库实现嘴巴和眼睛的分割。以下是基本步骤:
1. 使用dlib中的人脸检测器检测人脸。
2. 使用dlib中的关键点检测器检测人脸的关键点,其中包括眼睛和嘴巴的关键点。
3. 根据关键点的位置,使用OpenCV中的形态学操作和阈值处理来分割眼睛和嘴巴。
你可以使用以下代码片段开始:
```python
import cv2
import dlib
# 加载人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
# 读取图像
image = cv2.imread('test.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray, 0)
for face in faces:
# 检测关键点
landmarks = predictor(gray, face)
# 提取嘴巴关键点
mouth_points = []
for i in range(48, 68):
x = landmarks.part(i).x
y = landmarks.part(i).y
mouth_points.append((x, y))
# 提取左眼关键点
left_eye_points = []
for i in range(36, 42):
x = landmarks.part(i).x
y = landmarks.part(i).y
left_eye_points.append((x, y))
# 提取右眼关键点
right_eye_points = []
for i in range(42, 48):
x = landmarks.part(i).x
y = landmarks.part(i).y
right_eye_points.append((x, y))
# 分割嘴巴
mouth_mask = np.zeros((image.shape[0], image.shape[1]), dtype=np.uint8)
mouth_hull = cv2.convexHull(np.array(mouth_points))
cv2.drawContours(mouth_mask, [mouth_hull], -1, 255, -1)
mouth = cv2.bitwise_and(image, image, mask=mouth_mask)
# 分割左眼
left_eye_mask = np.zeros((image.shape[0], image.shape[1]), dtype=np.uint8)
left_eye_hull = cv2.convexHull(np.array(left_eye_points))
cv2.drawContours(left_eye_mask, [left_eye_hull], -1, 255, -1)
left_eye = cv2.bitwise_and(image, image, mask=left_eye_mask)
# 分割右眼
right_eye_mask = np.zeros((image.shape[0], image.shape[1]), dtype=np.uint8)
right_eye_hull = cv2.convexHull(np.array(right_eye_points))
cv2.drawContours(right_eye_mask, [right_eye_hull], -1, 255, -1)
right_eye = cv2.bitwise_and(image, image, mask=right_eye_mask)
# 显示结果
cv2.imshow('Mouth', mouth)
cv2.imshow('Left Eye', left_eye)
cv2.imshow('Right Eye', right_eye)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,此代码片段仅用于演示目的,并且可能需要进行更改以适应您的特定情况。
阅读全文
相关推荐
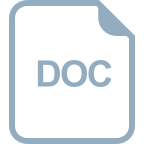
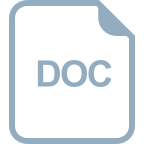
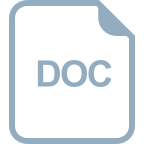


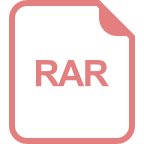
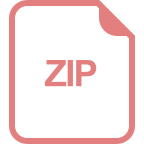
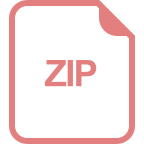
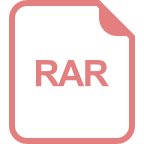
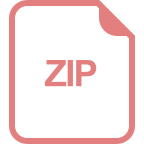
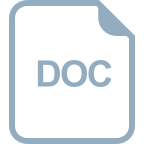
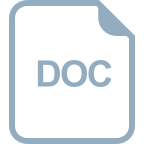
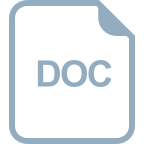
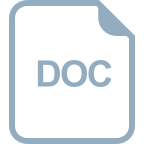
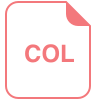
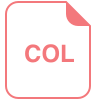
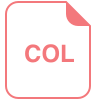
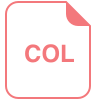
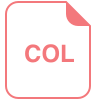