n Define a class Circle and derive the class Cylinder from the class Circle. n The specific requirements are as follows: l In the main function, input the radius of the circle and output the area of the corresponding circle. Then, input the height of the cylinder, output the surface area and volume of the corresponding cylinder.
时间: 2024-02-20 14:01:47 浏览: 83
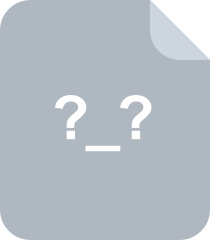
Designing with Data: Improving the User Experience with A/B Testing

Sure, here's an example implementation of the classes Circle and Cylinder in Python:
```python
import math
class Circle:
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
class Cylinder(Circle):
def __init__(self, radius, height):
super().__init__(radius)
self.height = height
def surface_area(self):
return 2 * math.pi * self.radius * self.height + 2 * super().area()
def volume(self):
return super().area() * self.height
# Example usage
radius = float(input("Enter the radius of the circle: "))
circle = Circle(radius)
print(f"The area of the circle is {circle.area()}")
height = float(input("Enter the height of the cylinder: "))
cylinder = Cylinder(radius, height)
print(f"The surface area of the cylinder is {cylinder.surface_area()}")
print(f"The volume of the cylinder is {cylinder.volume()}")
```
In the main function, we first create a `Circle` object with the given radius and compute its area using the `area` method. Then, we create a `Cylinder` object with the same radius and the given height. We can use the `surface_area` and `volume` methods of the `Cylinder` class to compute the surface area and volume of the cylinder, respectively, using the inherited `area` method of the `Circle` class.
Note that the `super()` function is used to call the methods of the parent class (`Circle`) in the `__init__`, `surface_area`, and `volume` methods of the `Cylinder` class. This allows us to avoid duplicating code and make use of the functionality already implemented in the `Circle` class.
阅读全文
相关推荐
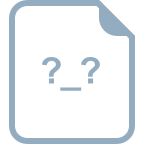
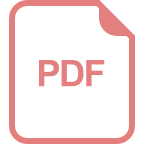
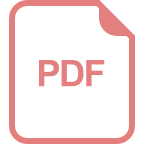
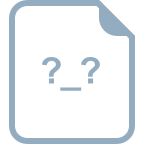
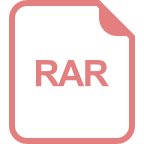
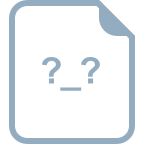
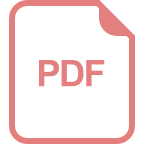
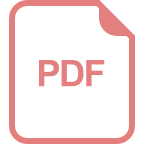
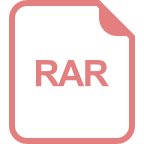
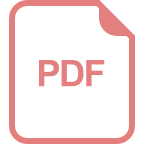
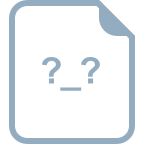
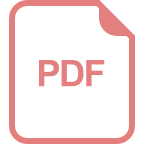
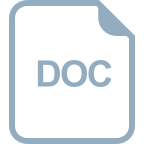