.model small .stack 100h .data msg db 'The string is a palindrome.$' msg1 db 'The string is a palindrome.$' msg2 db 'The string is not a palindrome.$' str db 'abccba$' .code main proc mov ax, @data mov ds, ax ; 计算字符串长度 mov si, 0 mov cx, 0 mov cl, 6 dec cx ; 双重循环判断回文字符串 mov di, cx shr cx, 1 mov bx, 0 cmp cx, bx jle palindrome mov bx, 1 outer_loop: mov al, [str+si+1] mov ah, [str+di+1] cmp al, ah jne not_palindrome inc si dec di cmp si, di jg palindrome inner_loop: mov al, [str+si+1] mov ah, [str+di+1] cmp al, ah jne not_palindrome inc si dec di cmp si, di jle inner_loop palindrome: ; 输出回文字符串信息 lea dx, msg1 mov ah, 09h int 21h jmp exit_program not_palindrome: ; 输出非回文字符串信息 lea dx, msg2 mov ah, 09h int 21h exit_program: mov ah, 4ch int 21h main endp end main为什么输出结果只有一次
时间: 2024-01-12 15:02:51 浏览: 23
在这个程序中,循环只会执行一次是因为程序在输出回文字符串信息或非回文字符串信息后,直接跳转到程序结束的地方,而没有给用户重新输入字符串的机会。因此,程序只会判断一次固定的字符串 `abccba$` 是否为回文字符串,并输出对应的信息。
如果你想要让程序能够多次判断字符串是否为回文字符串,可以在程序的结尾处添加一个循环,让用户可以多次输入字符串并进行判断。例如,可以使用以下代码实现:
```
.model small
.stack 100h
.data
msg db 'Enter a string (or press Enter to exit): $'
msg1 db 'The string is a palindrome.$'
msg2 db 'The string is not a palindrome.$'
str db 80h dup('$')
.code
main proc
mov ax, @data
mov ds, ax
input_loop:
; 输出提示信息
lea dx, msg
mov ah, 09h
int 21h
; 读取字符串
lea dx, str
mov ah, 0Ah
int 21h
; 如果用户按下了 Enter 键,退出程序
cmp byte ptr [str+2], 0Dh
je exit_program
; 计算字符串长度
mov si, 0
mov cx, 0
mov cl, [str+1]
dec cx
; 双重循环判断回文字符串
mov di, cx
shr cx, 1
mov bx, 0
cmp cx, bx
jle palindrome
mov bx, 1
outer_loop:
mov al, [str+si+2]
mov ah, [str+di+2]
cmp al, ah
jne not_palindrome
inc si
dec di
cmp si, di
jg palindrome
inner_loop:
mov al, [str+si+2]
mov ah, [str+di+2]
cmp al, ah
jne not_palindrome
inc si
dec di
cmp si, di
jle inner_loop
palindrome:
; 输出回文字符串信息
lea dx, msg1
mov ah, 09h
int 21h
jmp input_loop
not_palindrome:
; 输出非回文字符串信息
lea dx, msg2
mov ah, 09h
int 21h
jmp input_loop
exit_program:
mov ah, 4ch
int 21h
main endp
end main
```
在这个程序中,用户可以多次输入字符串,程序会重复执行字符串判断的部分,并输出对应的信息,直到用户按下 Enter 键结束程序。
相关推荐
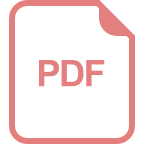
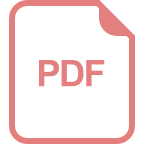







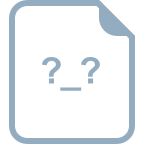
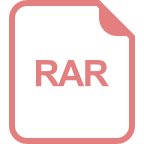
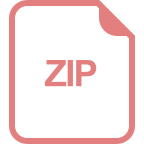
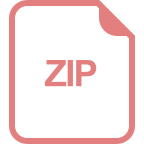
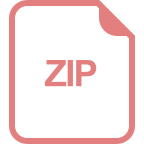
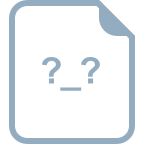