帮我写一段java代码,实现本地文件的非对称加密和解密
时间: 2024-03-13 14:44:25 浏览: 17
好的,以下是一个完整的 Java 代码示例,使用 RSA 算法实现本地文件的非对称加密和解密:
```java
import java.io.*;
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.*;
public class FileEncryption {
public static void main(String[] args) {
String inputFile = "input.txt"; // 待加密的文件名
String encryptedFile = "encrypted.enc"; // 加密后的文件名
String decryptedFile = "decrypted.txt"; // 解密后的文件名
String publicKeyFile = "public.key"; // 公钥文件名
String privateKeyFile = "private.key"; // 私钥文件名
try {
// 生成公钥和私钥
KeyPair keyPair = generateKeyPair();
// 将公钥和私钥写入文件
writeKeyToFile(publicKeyFile, keyPair.getPublic());
writeKeyToFile(privateKeyFile, keyPair.getPrivate());
// 读取公钥
PublicKey publicKey = readPublicKey(publicKeyFile);
// 读取私钥
PrivateKey privateKey = readPrivateKey(privateKeyFile);
// 加密文件
encryptFile(inputFile, encryptedFile, publicKey);
System.out.println("Encryption completed successfully.");
// 解密文件
decryptFile(encryptedFile, decryptedFile, privateKey);
System.out.println("Decryption completed successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
// 生成公钥和私钥
public static KeyPair generateKeyPair() throws NoSuchAlgorithmException {
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048);
return keyGen.generateKeyPair();
}
// 将公钥或私钥写入文件
public static void writeKeyToFile(String fileName, Key key) throws IOException {
FileOutputStream fos = new FileOutputStream(fileName);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(key);
oos.close();
}
// 读取公钥
public static PublicKey readPublicKey(String fileName) throws Exception {
InputStream in = new FileInputStream(fileName);
ObjectInputStream oin = new ObjectInputStream(new BufferedInputStream(in));
try {
PublicKey key = (PublicKey) oin.readObject();
return key;
} finally {
oin.close();
}
}
// 读取私钥
public static PrivateKey readPrivateKey(String fileName) throws Exception {
InputStream in = new FileInputStream(fileName);
ObjectInputStream oin = new ObjectInputStream(new BufferedInputStream(in));
try {
PrivateKey key = (PrivateKey) oin.readObject();
return key;
} finally {
oin.close();
}
}
// 加密文件
public static void encryptFile(String inputFile, String outputFile, PublicKey publicKey) throws Exception {
// 读取待加密的文件
byte[] input = readInputFile(inputFile);
// 创建Cipher对象,使用公钥进行加密
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
// 加密文件内容
byte[] encrypted = cipher.doFinal(input);
// 将加密后的内容写入到输出文件中
writeOutputFile(outputFile, encrypted);
}
// 解密文件
public static void decryptFile(String inputFile, String outputFile, PrivateKey privateKey) throws Exception {
// 读取待解密的文件
byte[] input = readInputFile(inputFile);
// 创建Cipher对象,使用私钥进行解密
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
// 解密文件内容
byte[] decrypted = cipher.doFinal(input);
// 将解密后的内容写入到输出文件中
writeOutputFile(outputFile, decrypted);
}
// 读取文件
public static byte[] readInputFile(String fileName) throws IOException {
File file = new File(fileName);
FileInputStream fis = new FileInputStream(file);
byte[] data = new byte[(int) file.length()];
fis.read(data);
fis.close();
return data;
}
// 写入文件
public static void writeOutputFile(String fileName, byte[] data) throws IOException {
FileOutputStream fos = new FileOutputStream(fileName);
fos.write(data);
fos.close();
}
}
```
在上面的代码中,我们生成了公钥和私钥,并将它们写入文件中。然后,我们使用公钥对文件进行加密,使用私钥对加密后的文件进行解密。最终,我们可以得到原始的文件内容。
需要注意的是,本示例代码中使用了 PKCS1Padding 填充方式,如果需要使用其他填充方式,可以根据实际情况修改代码。此外,实际应用中,我们还需要考虑很多细节,如密钥管理、加密算法的选择、加密模式、填充方式等。
相关推荐
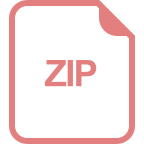
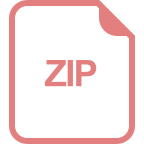
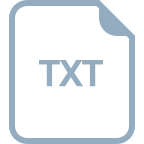














