六自由度串联机器人代码
时间: 2023-11-12 19:53:25 浏览: 218
对于六自由度串联机器人的代码,可以根据具体的机器人型号和控制系统来编写。下面是一个示例代码,用于控制六自由度串联机器人的运动:
```python
import numpy as np
import math
# 机器人参数
L1 = 1.0 # 第一段臂长
L2 = 1.0 # 第二段臂长
L3 = 1.0 # 第三段臂长
# 正向运动学
def forward_kinematics(theta):
x = L1 * math.cos(theta[0]) + L2 * math.cos(theta[0] + theta[1]) + L3 * math.cos(theta[0] + theta[1] + theta[2])
y = L1 * math.sin(theta[0]) + L2 * math.sin(theta[0] + theta[1]) + L3 * math.sin(theta[0] + theta[1] + theta[2])
z = theta[3] + theta[4] + theta[5]
return [x, y, z]
# 逆向运动学
def inverse_kinematics(position):
x, y, z = position[0], position[1], position[2]
theta3 = z - theta[0] - theta[1]
c2 = (x**2 + y**2 - L1**2 - L2**2) / (2 * L1 * L2)
s2 = math.sqrt(1 - c2**2)
theta2 = math.atan2(s2, c2)
c1 = (L1 + L2 * c2) * x + L2 * s2 * y
s1 = (L1 + L2 * c2) * y - L2 * s2 * x
theta1 = math.atan2(s1, c1)
return [theta1, theta2, theta3]
# 控制器
def controller(desired_position, current_position):
error = np.array(desired_position) - np.array(current_position)
# 根据误差计算控制指令
# ...
return control_command
# 主循环
def main():
desired_position = [1.0, 2.0, 3.0] # 期望位置
current_position = forward_kinematics([0.0, 0.0, 0.0]) # 当前位置
while True:
control_command = controller(desired_position, current_position)
# 发送控制指令给机器人
# ...
# 更新当前位置
current_position = forward_kinematics([theta1, theta2, theta3])
# 检查是否达到期望位置
if np.linalg.norm(np.array(desired_position) - np.array(current_position)) < 0.01:
break
if __name__ == "__main__":
main()
```
请注意,这只是一个简单的示例代码,实际的六自由度串联机器人控制可能需要根据具体情况进行调整和扩展。此外,在实际应用中可能还需要考虑安全性、碰撞检测等其他因素。
阅读全文
相关推荐
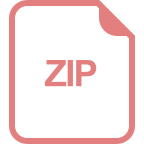
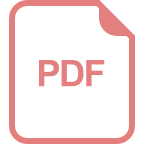
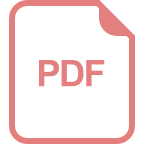
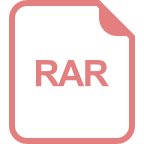
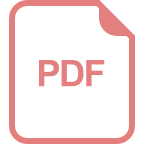
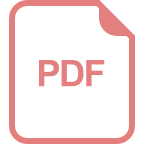
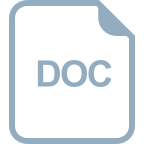
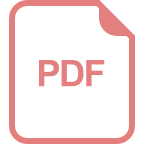
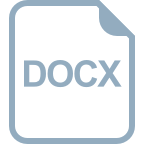
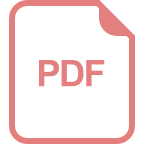
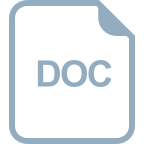
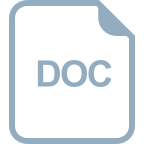
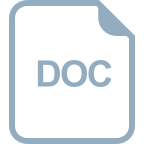