C++11 thread的所有用法 讲解
时间: 2023-09-09 13:06:09 浏览: 197
C11标准引入了一套线程库,包括了以下几个头文件:
- `<threads.h>`:包含了线程相关函数和类型的声明,例如`thrd_t`、`mtx_t`、`cnd_t`、`thrd_create()`、`mtx_lock()`等。
- `<stdatomic.h>`:包含了原子操作相关的函数和类型声明,例如`atomic_int`、`atomic_fetch_add()`等。
- `<time.h>`:包含了时间相关函数和类型声明,例如`timespec`、`nanosleep()`等。
下面是C11线程库的几个主要部分:
### 线程管理
- `thrd_t`:线程对象,可用于创建、等待和销毁线程。
- `thrd_create()`:创建新线程。
- `thrd_join()`:等待线程结束。
- `thrd_detach()`:分离线程,使其运行在后台。
- `thrd_sleep()`:线程休眠一段时间。
```c
#include <stdio.h>
#include <threads.h>
int thread_func(void* arg) {
printf("Hello, world!\n");
return 0;
}
int main() {
thrd_t thread;
thrd_create(&thread, thread_func, NULL);
thrd_join(thread, NULL);
return 0;
}
```
### 互斥量
- `mtx_t`:互斥量对象,用于保护共享资源。
- `mtx_init()`:初始化互斥量。
- `mtx_lock()`:锁定互斥量。
- `mtx_unlock()`:解锁互斥量。
- `mtx_destroy()`:销毁互斥量。
```c
#include <stdio.h>
#include <threads.h>
mtx_t mutex;
void* thread_func(void* arg) {
mtx_lock(&mutex);
printf("Thread %d locked the mutex.\n", *(int*)arg);
mtx_unlock(&mutex);
return NULL;
}
int main() {
mtx_init(&mutex, mtx_plain);
thrd_t thread1, thread2;
int arg1 = 1, arg2 = 2;
thrd_create(&thread1, thread_func, &arg1);
thrd_create(&thread2, thread_func, &arg2);
thrd_join(thread1, NULL);
thrd_join(thread2, NULL);
mtx_destroy(&mutex);
return 0;
}
```
### 条件变量
- `cnd_t`:条件变量对象,用于等待和通知条件。
- `cnd_init()`:初始化条件变量。
- `cnd_wait()`:等待条件变量满足。
- `cnd_signal()`:通知条件变量满足。
- `cnd_broadcast()`:通知所有等待条件变量的线程。
```c
#include <stdio.h>
#include <threads.h>
mtx_t mutex;
cnd_t cond;
void* thread_func(void* arg) {
mtx_lock(&mutex);
printf("Thread %d locked the mutex.\n", *(int*)arg);
cnd_wait(&cond, &mutex);
printf("Thread %d received the signal.\n", *(int*)arg);
mtx_unlock(&mutex);
return NULL;
}
int main() {
mtx_init(&mutex, mtx_plain);
cnd_init(&cond);
thrd_t thread1, thread2;
int arg1 = 1, arg2 = 2;
thrd_create(&thread1, thread_func, &arg1);
thrd_create(&thread2, thread_func, &arg2);
thrd_sleep(&(struct timespec){.tv_sec=1}, NULL);
cnd_signal(&cond);
thrd_sleep(&(struct timespec){.tv_sec=1}, NULL);
cnd_broadcast(&cond);
thrd_join(thread1, NULL);
thrd_join(thread2, NULL);
mtx_destroy(&mutex);
cnd_destroy(&cond);
return 0;
}
```
### 原子操作
C11标准引入了一套原子操作函数,可用于对共享变量进行原子操作,避免了多线程竞争的问题。
```c
#include <stdio.h>
#include <stdatomic.h>
#include <threads.h>
atomic_int counter = ATOMIC_VAR_INIT(0);
void* thread_func(void* arg) {
for (int i = 0; i < 1000000; ++i) {
atomic_fetch_add(&counter, 1);
}
return NULL;
}
int main() {
thrd_t thread1, thread2;
thrd_create(&thread1, thread_func, NULL);
thrd_create(&thread2, thread_func, NULL);
thrd_join(thread1, NULL);
thrd_join(thread2, NULL);
printf("Counter value: %d\n", counter);
return 0;
}
```
以上就是C11线程库的主要内容。需要注意的是,C11线程库的兼容性并不好,不同的编译器可能会有不同的实现。
阅读全文
相关推荐
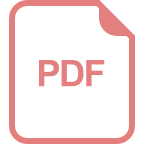
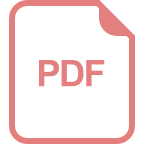
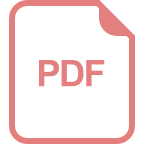

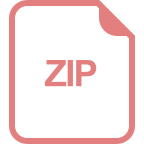
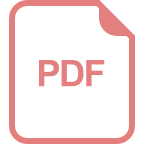
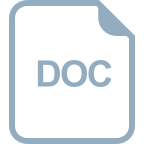
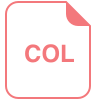
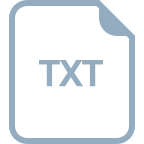
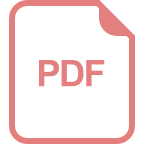
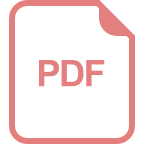
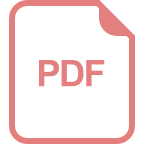
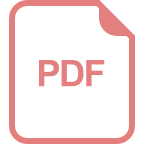
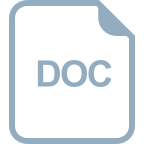
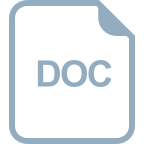
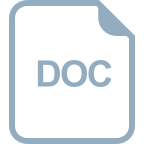
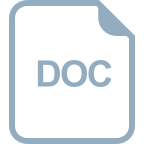
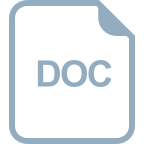
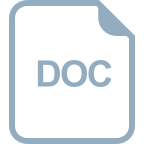