C++ thread的所有用法 讲解
时间: 2023-09-08 18:10:17 浏览: 212
C语言中的线程是一种轻量级的进程,它可以在同一个进程中并发执行多个任务。线程可以共享进程的资源,如内存空间、文件句柄等,但每个线程都拥有自己的寄存器和栈空间。线程的使用可以提高程序的运行效率,增强程序的并发性能。
以下是C语言中线程的常用用法:
1. 创建线程
创建线程需要使用pthread_create()函数。它的原型如下:
```
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void*), void *arg);
```
参数说明:
- thread:指向线程标识符的指针。
- attr:线程属性,通常为NULL。
- start_routine:线程函数的指针。
- arg:传递给线程函数的参数。
示例代码:
```
#include <pthread.h>
#include <stdio.h>
void* thread_function(void* arg)
{
printf("Thread is running.\n");
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
pthread_create(&tid, NULL, thread_function, NULL);
printf("Thread created.\n");
pthread_join(tid, NULL);
printf("Thread finished.\n");
return 0;
}
```
2. 终止线程
线程可以通过调用pthread_exit()函数来终止自己。如果一个线程已经被终止,其他线程可以通过pthread_join()函数来等待它的结束。如果一个线程不希望其他线程等待它的结束,可以使用pthread_detach()函数将它分离。
示例代码:
```
#include <pthread.h>
#include <stdio.h>
void* thread_function(void* arg)
{
printf("Thread is running.\n");
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
pthread_create(&tid, NULL, thread_function, NULL);
printf("Thread created.\n");
pthread_join(tid, NULL);
printf("Thread finished.\n");
return 0;
}
```
3. 线程同步
在多线程编程中,经常需要进行线程同步,以保证各个线程之间的顺序和正确性。C语言提供了多种线程同步的机制,如互斥锁、条件变量、信号量等。
互斥锁:在多个线程访问共享资源时,需要保证同时只有一个线程能够访问,这时就需要使用互斥锁来保护共享资源。
示例代码:
```
#include <pthread.h>
#include <stdio.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int counter = 0;
void* thread_function(void* arg)
{
int i;
for (i = 0; i < 100000; i++) {
pthread_mutex_lock(&mutex);
counter++;
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
int main()
{
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, thread_function, NULL);
pthread_create(&tid2, NULL, thread_function, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
printf("Counter value: %d\n", counter);
return 0;
}
```
条件变量:条件变量用于在多个线程之间传递信号,以便线程之间能够相互通信。
示例代码:
```
#include <pthread.h>
#include <stdio.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
int counter = 0;
void* thread_function(void* arg)
{
pthread_mutex_lock(&mutex);
while (counter < 3) {
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
printf("Thread finished.\n");
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
pthread_create(&tid, NULL, thread_function, NULL);
pthread_mutex_lock(&mutex);
counter++;
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
pthread_mutex_lock(&mutex);
counter++;
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
pthread_mutex_lock(&mutex);
counter++;
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
pthread_join(tid, NULL);
printf("All threads finished.\n");
return 0;
}
```
信号量:信号量用于在多个线程之间同步访问共享资源。
示例代码:
```
#include <pthread.h>
#include <semaphore.h>
#include <stdio.h>
sem_t sem;
int counter = 0;
void* thread_function(void* arg)
{
int i;
for (i = 0; i < 100000; i++) {
sem_wait(&sem);
counter++;
sem_post(&sem);
}
pthread_exit(NULL);
}
int main()
{
pthread_t tid1, tid2;
sem_init(&sem, 0, 1);
pthread_create(&tid1, NULL, thread_function, NULL);
pthread_create(&tid2, NULL, thread_function, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
sem_destroy(&sem);
printf("Counter value: %d\n", counter);
return 0;
}
```
4. 线程池
线程池是一种常用的线程管理方法,它可以预先创建一些线程,并将它们保存在一个线程池中,以便随时调用。这种方法可以避免频繁地创建和销毁线程,提高程序的效率。
示例代码:
```
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#define THREAD_POOL_SIZE 5
typedef struct {
pthread_t thread_id;
int busy;
} thread_t;
thread_t thread_pool[THREAD_POOL_SIZE];
void* thread_function(void* arg)
{
int index = *(int*)arg;
printf("Thread %d is running.\n", index);
while (1) {
// do something
thread_pool[index].busy = 0;
}
pthread_exit(NULL);
}
int main()
{
int i;
for (i = 0; i < THREAD_POOL_SIZE; i++) {
pthread_create(&thread_pool[i].thread_id, NULL, thread_function, &i);
}
while (1) {
// do something
for (i = 0; i < THREAD_POOL_SIZE; i++) {
if (!thread_pool[i].busy) {
thread_pool[i].busy = 1;
break;
}
}
}
return 0;
}
```
以上是C语言中线程的常用用法。在实际使用中,需要根据具体情况选择合适的线程同步方法,以保证程序的正确性和效率。
阅读全文
相关推荐
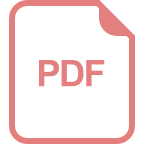

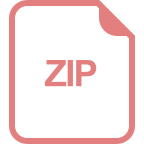
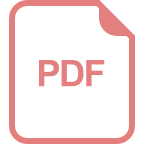
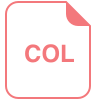
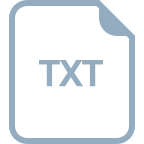
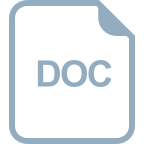
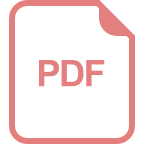
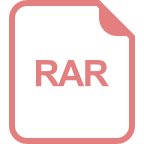
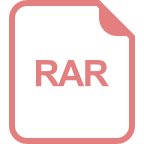
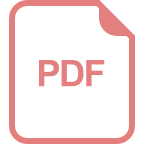
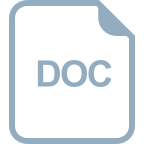
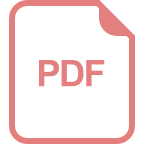
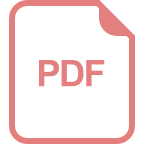
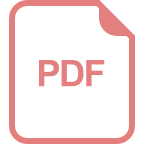
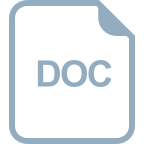
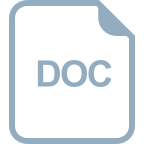