编写求阶乘的函数,在主函数中调用该函数,求n!并输出结果,n从键盘上输入(n<10)。使用c++
时间: 2023-06-14 07:08:26 浏览: 166
以下是使用C++编写的求阶乘的函数及主函数示例代码:
```c++
#include <iostream>
using namespace std;
int factorial(int n) {
if (n == 0 || n == 1) { // 0!和1!的值都为1
return 1;
} else {
return n * factorial(n-1); // 递归调用自身
}
}
int main() {
int n;
cout << "请输入一个小于10的整数n:" << endl;
cin >> n;
cout << n << "! = " << factorial(n) << endl;
return 0;
}
```
该程序先定义了一个函数`factorial`,用于求n的阶乘。若n为0或1,则直接返回1;否则,递归调用自身,计算n * (n-1) * (n-2) * … * 2 * 1的值。
在主函数中,用户从键盘上输入一个小于10的整数n,然后调用`factorial`函数求解n的阶乘,并输出结果。
相关问题
编写求阶乘的函数,在主函数中调用该函数,求n!并输出结果,n从键盘上输入(n<10)
以下是使用递归方式求解阶乘的函数代码:
```c++
#include <iostream>
using namespace std;
int factorial(int n) {
if (n == 1 || n == 0) {
return 1;
} else {
return n * factorial(n-1);
}
}
int main() {
int n;
cout << "请输入n的值(n<10):" << endl;
cin >> n;
cout << n << "! = " << factorial(n) << endl;
return 0;
}
```
该程序中,`factorial()` 函数使用了递归方式求解阶乘。当输入的 n 等于 1 或 0 时,递归停止,返回 1;否则,继续递归调用 `factorial()` 函数。
在 `main()` 函数中,程序从键盘输入 n 的值,然后调用 `factorial()` 函数求解 n! 并输出结果。
需要注意的是,由于 n 的值较小,因此在使用递归方式求解阶乘时不会出现栈溢出的情况。如果需要求解较大的阶乘,建议使用循环方式实现。
用C++编写函数power (),实现求N!在主函数中输入N,并调用该函数,输出结果
在C++中,你可以通过递归或者循环的方式来实现计算阶乘的power()函数。这里我们先来看一个简单的递归版本:
```cpp
#include <iostream>
// 动态规划优化的递归函数,用于计算阶乘
unsigned long long factorial(unsigned int n) {
if (n == 0 || n == 1)
return 1; // 阶乘的基本情况,0和1的阶乘都是1
else
return n * factorial(n - 1); // 递归调用,n! = n * (n - 1)!
}
int main() {
unsigned int N;
std::cout << "请输入一个非负整数N: ";
std::cin >> N;
// 检查输入是否合法
if (N < 0) {
std::cerr << "错误:阶乘只对非负整数定义。" << std::endl;
return 1;
}
unsigned long long result = factorial(N);
std::cout << "N!的结果是: " << result << std::endl;
return 0;
}
```
在这个程序中,`factorial()`函数负责计算阶乘,`main()`函数则处理用户输入并调用`factorial()`。请注意,由于C++默认整型溢出可能会导致错误,对于较大的数值,可能需要使用`unsigned long long`作为返回类型。
阅读全文
相关推荐
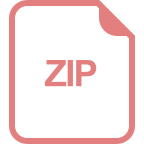
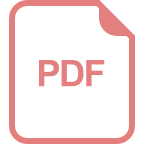
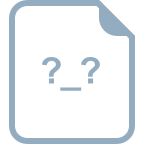













