jaxb解析xml 数组
时间: 2023-09-28 08:04:00 浏览: 51
若要使用JAXB解析XML数组,需要按照以下步骤进行操作:
1. 创建一个包含所有要解析的数组元素的Java类。
2. 在该类上使用`@XmlRootElement`注释,指定XML的根元素名称。
3. 在该类中使用`@XmlElement`注释,指定XML中数组元素的名称。
4. 在解析XML时,使用JAXB的`unmarshal()`方法将XML转换为Java对象。
5. 将返回的Java对象转换为数组。
以下是一个示例Java类,用于解析包含`book`元素的XML数组:
```
@XmlRootElement(name = "books")
public class Books {
@XmlElement(name = "book")
private List<Book> books;
public List<Book> getBooks() {
return books;
}
public void setBooks(List<Book> books) {
this.books = books;
}
}
public class Book {
private String title;
private String author;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
```
在上面的示例中,`Books`类包含一个名为`books`的`List`,其中每个元素都是`Book`对象。`Books`类上使用了`@XmlRootElement`注释,指定XML中的根元素名称为`books`。`Book`类中没有使用任何JAXB注释,因为不需要指定XML元素的名称。
以下是一个示例XML文件,其中包含两个`book`元素:
```
<books>
<book>
<title>Java Programming</title>
<author>John Smith</author>
</book>
<book>
<title>Python Programming</title>
<author>Jane Doe</author>
</book>
</books>
```
要解析该XML文件,可以使用以下代码:
```
File file = new File("books.xml");
JAXBContext jaxbContext = JAXBContext.newInstance(Books.class);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
Books books = (Books) jaxbUnmarshaller.unmarshal(file);
List<Book> bookList = books.getBooks();
```
在上面的代码中,首先使用`JAXBContext`创建一个`Unmarshaller`对象。然后,使用`unmarshal()`方法将XML文件转换为`Books`对象。最后,使用`getBooks()`方法获取`Books`对象中的`List`,它包含所有的`Book`对象。
相关推荐
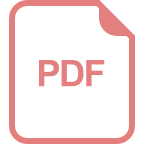
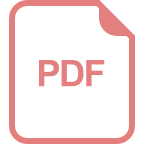
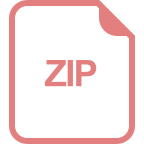
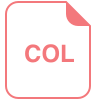
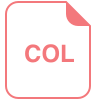
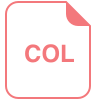











