参考附录1-4,选择数据库新建一个表,新建程序可以访问、插入、更新这个表。(注意,附录2-4没有将数据库访问信息直接写在程序中,而是将信息写在同一个工程中的 properties 文件)
时间: 2024-03-05 14:53:42 浏览: 82
好的,根据您的要求,我可以为您提供以下步骤:
1. 打开您的数据库管理工具,并登录您的数据库。
2. 在数据库中创建一个新的表,可以使用 SQL 语句创建,例如:
```
CREATE TABLE my_table (
id INT PRIMARY KEY,
name VARCHAR(50),
age INT
);
```
这个语句将创建一个名为 my_table 的表,包含三个列:id、name 和 age。
3. 在您的程序中,使用 JDBC 连接到您的数据库,并访问新创建的表。您可以在 properties 文件中设置数据库连接信息,例如:
```
db.url=jdbc:mysql://localhost:3306/my_database
db.user=my_username
db.password=my_password
```
在程序中,您可以使用以下代码读取这些信息:
```
Properties props = new Properties();
InputStream in = new FileInputStream("path/to/your/properties/file");
props.load(in);
String url = props.getProperty("db.url");
String user = props.getProperty("db.user");
String password = props.getProperty("db.password");
Connection conn = DriverManager.getConnection(url, user, password);
```
这会使用您在 properties 文件中设置的信息创建一个 JDBC 连接。
4. 在程序中,使用 SQL 语句向新创建的表中插入数据,例如:
```
String sql = "INSERT INTO my_table (id, name, age) VALUES (?, ?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, 1);
stmt.setString(2, "John");
stmt.setInt(3, 30);
stmt.executeUpdate();
```
这将向 my_table 表中插入一行数据,包含 id 为 1、name 为 John、age 为 30。
5. 在程序中,使用 SQL 语句更新新创建的表中的数据,例如:
```
String sql = "UPDATE my_table SET age = ? WHERE name = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, 31);
stmt.setString(2, "John");
stmt.executeUpdate();
```
这将更新 my_table 表中 name 为 John 的行的 age 值为 31。
希望这些步骤可以帮助您创建一个程序,可以访问、插入、更新您的新表。如果您有任何问题,请随时问我。
阅读全文
相关推荐
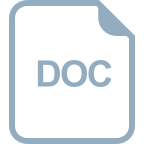

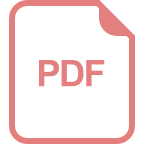
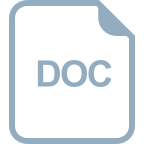
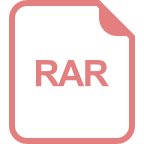
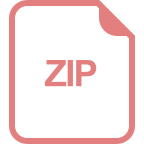
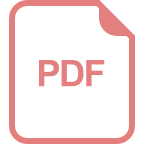
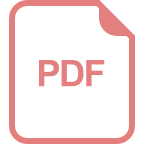
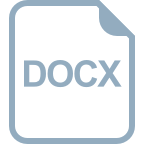
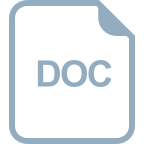
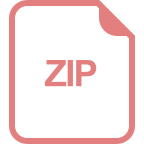
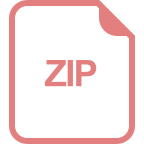
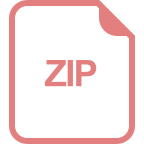
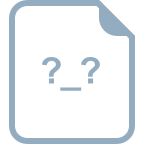
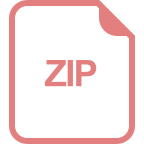