Java用高级语言编写和调试一个简单的银行家算法程序。用银行家算法实现资源分配。
时间: 2024-02-17 22:59:46 浏览: 91
以下是一个简单的Java实现银行家算法的程序,实现了资源分配的功能:
```java
import java.util.Scanner;
public class BankerAlgorithm {
// 定义全局变量,表示系统中每种资源的总量
static int[] resources = {10, 5, 7};
// 定义全局变量,表示每个进程已经分配的资源数量
static int[][] allocation = {
{0, 1, 0},
{2, 0, 0},
{3, 0, 2},
{2, 1, 1},
{0, 0, 2}
};
// 定义全局变量,表示每个进程还需要的资源数量
static int[][] need = {
{7, 4, 7},
{0, 1, 0},
{0, 3, 2},
{1, 3, 4},
{3, 3, 0}
};
// 定义全局变量,表示每种资源还剩余的数量
static int[] available = {2, 1, 3};
// 定义函数,判断是否可以分配资源
public static boolean check(int process, int[] request) {
for (int i = 0; i < resources.length; i++) {
if (request[i] > need[process][i] || request[i] > available[i]) {
return false;
}
}
return true;
}
// 定义函数,执行分配资源
public static void allocate(int process, int[] request) {
for (int i = 0; i < resources.length; i++) {
allocation[process][i] += request[i];
need[process][i] -= request[i];
available[i] -= request[i];
}
}
// 定义函数,执行释放资源
public static void release(int process, int[] release) {
for (int i = 0; i < resources.length; i++) {
allocation[process][i] -= release[i];
need[process][i] += release[i];
available[i] += release[i];
}
}
// 定义函数,判断系统是否处于安全状态
public static boolean isSafe() {
boolean[] finish = new boolean[allocation.length];
int[] work = available.clone();
while (true) {
boolean flag = false;
for (int i = 0; i < allocation.length; i++) {
if (!finish[i] && check(i, need[i])) {
flag = true;
finish[i] = true;
for (int j = 0; j < resources.length; j++) {
work[j] += allocation[i][j];
}
}
}
if (!flag) {
break;
}
}
for (boolean f : finish) {
if (!f) {
return false;
}
}
return true;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (true) {
// 输出当前系统的状态
System.out.println("当前系统状态:");
System.out.println("资源总量:" + java.util.Arrays.toString(resources));
System.out.println("每个进程已经分配的资源数量:");
for (int[] a : allocation) {
System.out.println(java.util.Arrays.toString(a));
}
System.out.println("每个进程还需要的资源数量:");
for (int[] n : need) {
System.out.println(java.util.Arrays.toString(n));
}
System.out.println("每种资源还剩余的数量:" + java.util.Arrays.toString(available));
// 输入要执行的操作
System.out.println("请输入要执行的操作(1表示申请资源,2表示释放资源,3表示退出):");
int op = sc.nextInt();
if (op == 1) {
// 输入要申请资源的进程编号和数量
System.out.println("请输入要申请资源的进程编号和数量(如:0 2 3 表示进程0要申请2个资源1和3个资源2):");
int process = sc.nextInt();
int[] request = new int[resources.length];
for (int i = 0; i < resources.length; i++) {
request[i] = sc.nextInt();
}
// 判断是否可以分配资源
if (check(process, request)) {
// 执行分配资源
allocate(process, request);
// 判断系统是否处于安全状态
if (isSafe()) {
System.out.println("分配成功,系统仍处于安全状态!");
} else {
// 如果不安全,撤销分配的资源
release(process, request);
System.out.println("分配失败,系统处于不安全状态,已撤销分配的资源!");
}
} else {
System.out.println("分配失败,申请资源数量超过了进程还需要的数量或超过了系统还剩余的数量!");
}
} else if (op == 2) {
// 输入要释放资源的进程编号和数量
System.out.println("请输入要释放资源的进程编号和数量(如:0 2 3 表示进程0要释放2个资源1和3个资源2):");
int process = sc.nextInt();
int[] release = new int[resources.length];
for (int i = 0; i < resources.length; i++) {
release[i] = sc.nextInt();
}
// 执行释放资源
release(process, release);
System.out.println("释放成功,系统处于安全状态!");
} else if (op == 3) {
// 退出程序
System.out.println("程序已退出!");
break;
} else {
System.out.println("无效的操作,请重新输入!");
}
}
sc.close();
}
}
```
该程序中,定义了全局变量 `resources`、`allocation`、`need` 和 `available`,用于表示系统中每种资源的总量、每个进程已经分配的资源数量、每个进程还需要的资源数量和每种资源还剩余的数量。
同时,定义了函数 `check`、`allocate`、`release` 和 `isSafe`,分别用于判断是否可以分配资源、执行分配资源、执行释放资源和判断系统是否处于安全状态。
在 `main` 函数中,通过循环实现不断地输入操作,并根据输入的操作执行相应的函数操作。其中,输入操作的代码使用了 `Scanner` 类,可以方便地从控制台输入数字和字符串。
运行该程序后,可以不断地输入操作,观察系统状态的变化,并了解银行家算法的原理和应用。
阅读全文
相关推荐
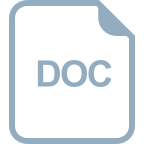
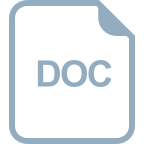
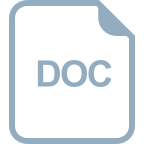
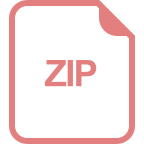
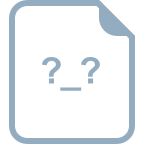
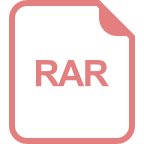
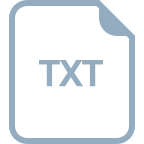
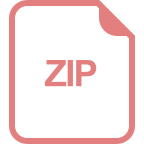
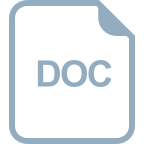
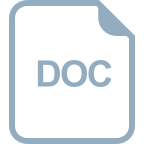
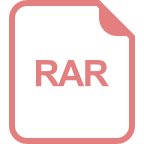
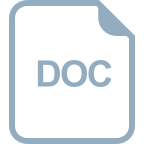
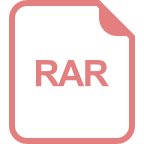
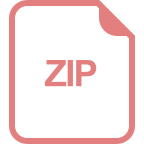
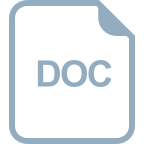
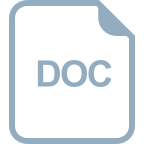
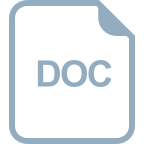
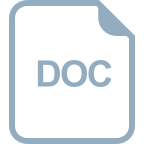
