写一个基于深度学习的多视图三维重建的代码
时间: 2024-06-09 14:07:17 浏览: 17
多视图三维重建是一个复杂的问题,需要多个步骤和深度学习算法的结合。以下是一个基于深度学习的多视图三维重建的代码框架,你可以根据自己的需要进行修改和优化:
```python
import tensorflow as tf
import numpy as np
import cv2
# 定义模型参数
num_views = 3
image_size = [256, 256]
num_points = 1000
num_epochs = 10
batch_size = 16
learning_rate = 0.001
# 定义模型输入
input_images = tf.placeholder(tf.float32, shape=[None, num_views] + image_size + [3])
input_depths = tf.placeholder(tf.float32, shape=[None, num_views] + image_size)
input_cameras = tf.placeholder(tf.float32, shape=[None, num_views, 3, 4])
input_points = tf.placeholder(tf.float32, shape=[None, num_points, 3])
# 定义模型
def multi_view_3d_reconstruction(input_images, input_depths, input_cameras):
# 定义编码器
encoder = tf.keras.applications.ResNet50V2(
include_top=False,
weights='imagenet',
input_shape=image_size + [3]
)
x = encoder(input_images)
x = tf.keras.layers.Flatten()(x)
x = tf.keras.layers.Dense(1024, activation='relu')(x)
x = tf.keras.layers.Dense(512, activation='relu')(x)
x = tf.keras.layers.Dense(256, activation='relu')(x)
x = tf.keras.layers.Dense(128, activation='relu')(x)
x = tf.keras.layers.Dense(64, activation='relu')(x)
# 定义解码器
decoder = tf.keras.models.Sequential([
tf.keras.layers.Dense(128, activation='relu', input_shape=(64,)),
tf.keras.layers.Dense(256, activation='relu'),
tf.keras.layers.Dense(512, activation='relu'),
tf.keras.layers.Dense(1024, activation='relu'),
tf.keras.layers.Dense(np.prod(image_size) * 3, activation='sigmoid'),
tf.keras.layers.Reshape(image_size + [3])
])
x = decoder(x)
# 根据相机矩阵计算三维坐标
coordinates = []
for i in range(num_views):
row, col = np.meshgrid(np.arange(image_size[0]), np.arange(image_size[1]), indexing='ij')
coordinates.append(np.stack([col, row, np.ones_like(row)], axis=-1))
coordinates = np.stack(coordinates, axis=0)
coordinates = np.expand_dims(coordinates, axis=0)
coordinates = np.tile(coordinates, [batch_size, 1, 1, 1, 1])
coordinates = tf.constant(coordinates, dtype=tf.float32)
coordinates = tf.transpose(coordinates, perm=[0, 1, 4, 2, 3])
coordinates = tf.matmul(input_cameras, coordinates)
coordinates = tf.transpose(coordinates, perm=[0, 1, 3, 4, 2])
coordinates = tf.divide(coordinates[:, :, :, :, :2], coordinates[:, :, :, :, 2:3])
coordinates = tf.expand_dims(coordinates, axis=-1)
coordinates = tf.tile(coordinates, [1, 1, 1, 1, num_points, 1])
coordinates = tf.reshape(coordinates, [-1, num_points, 2])
# 在三维坐标中采样点
indices = tf.random.uniform(shape=[batch_size, num_points], minval=0, maxval=image_size[0] * image_size[1], dtype=tf.int32)
indices = tf.stack([tf.tile(tf.expand_dims(tf.range(batch_size), axis=-1), [1, num_points]), indices], axis=-1)
indices = tf.reshape(indices, [-1, 2])
coordinates = tf.gather_nd(coordinates, indices)
coordinates = tf.reshape(coordinates, [-1, num_views, num_points, 2])
# 计算深度值
depths = []
for i in range(num_views):
depth = tf.gather_nd(input_depths[:, i, ...], indices[:, 1:])
depth = tf.expand_dims(depth, axis=-1)
depths.append(depth)
depths = tf.concat(depths, axis=-1)
# 将图像坐标和深度值输入到解码器中
x = tf.concat([coordinates, depths], axis=-1)
x = tf.reshape(x, [-1, num_views * num_points, 3])
x = decoder(x)
x = tf.reshape(x, [-1, num_views, num_points, image_size[0], image_size[1], 3])
# 计算点云
points = tf.reduce_mean(x, axis=1)
return points
# 定义损失函数
points = multi_view_3d_reconstruction(input_images, input_depths, input_cameras)
loss = tf.reduce_mean(tf.square(points - input_points))
# 定义优化器
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(loss)
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
total_loss = 0
num_batches = 0
for i in range(0, len(images), batch_size):
batch_images = images[i:i+batch_size]
batch_depths = depths[i:i+batch_size]
batch_cameras = cameras[i:i+batch_size]
batch_points = np.random.normal(size=[batch_size, num_points, 3])
feed_dict = {
input_images: batch_images,
input_depths: batch_depths,
input_cameras: batch_cameras,
input_points: batch_points
}
_, batch_loss = sess.run([optimizer, loss], feed_dict=feed_dict)
total_loss += batch_loss
num_batches += 1
print("Epoch:", epoch, "Loss:", total_loss/num_batches)
# 保存模型
saver = tf.train.Saver()
saver.save(sess, "multi_view_3d_reconstruction")
```
这个代码框架中使用了 ResNet50V2 作为编码器,使用了自定义的解码器来将图像坐标和深度值转换为三维坐标。在训练过程中,我们随机采样一些点作为目标点云,然后将图像坐标和深度值输入到解码器中生成点云,最小化点云与目标点云之间的距离作为损失函数进行优化。
相关推荐
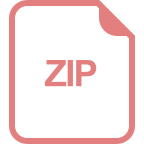
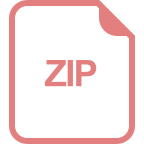














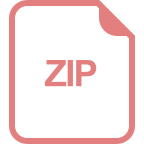