利用pytorch平台实现数字图像识别任务。 1、 获取数字图像数据集; 2、 搭建深度卷积神经网络; 3、 定义各种超参数,损失函数以及优化算法; 4、 网络训练; 5、 性能测试。
时间: 2023-12-14 20:05:35 浏览: 87
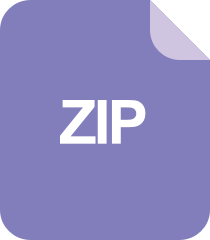
将使用Pytorch框架实现经典的图像分类网络、目标检测网络、图像分割网络,图像生成网络等,并会持续更新!!!.zip
好的,我可以帮你讲解一下利用PyTorch平台实现数字图像识别任务的步骤。
1. 获取数字图像数据集
首先,我们需要获取一个适合数字图像识别任务的数据集。常见的数字图像数据集包括MNIST、CIFAR-10、Fashion-MNIST等。其中,MNIST数据集是最简单的数据集之一,它包含了手写数字0到9的灰度图像,每张图像的大小为28x28像素。你可以通过下载官方提供的数据集或使用PyTorch内置的数据集来获取数据。
2. 搭建深度卷积神经网络
接下来,我们需要搭建一个深度卷积神经网络来进行数字图像识别。在PyTorch中,我们可以通过继承`nn.Module`类来构建网络。具体而言,我们需要定义网络的结构和前向传播函数。一个简单的卷积神经网络结构如下:
```
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
# 定义卷积层和池化层
self.conv1 = nn.Conv2d(1, 32, 3, 1)
self.conv2 = nn.Conv2d(32, 64, 3, 1)
self.dropout1 = nn.Dropout2d(0.25)
self.dropout2 = nn.Dropout2d(0.5)
self.fc1 = nn.Linear(9216, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = self.dropout1(x)
x = torch.flatten(x, 1)
x = F.relu(self.fc1(x))
x = self.dropout2(x)
x = self.fc2(x)
output = F.log_softmax(x, dim=1)
return output
```
这个网络包含两个卷积层、两个池化层、两个dropout层和两个全连接层。其中,第一个卷积层的输入通道数为1,输出通道数为32,卷积核大小为3x3,步长为1,并使用ReLU激活函数;第二个卷积层的输入通道数为32,输出通道数为64,其余与第一个卷积层相同。接下来是两个池化层,每个池化层的大小为2x2。之后是两个dropout层,分别设置为0.25和0.5,用于防止过拟合。最后是两个全连接层,分别将输出大小降至128和10(在MNIST数据集中,一共有10个类别)。
3. 定义各种超参数、损失函数和优化算法
在训练神经网络时,我们需要为其指定各种超参数,包括学习率、训练轮数、批次大小等。同时,我们需要定义一个损失函数来衡量网络输出与真实标签之间的差异,常见的损失函数包括交叉熵损失函数、均方误差损失函数等。最后,我们还需要选择一个优化算法来更新网络参数,常见的优化算法包括随机梯度下降(SGD)、Adam等。
```
# 定义超参数
learning_rate = 0.001
num_epochs = 5
batch_size = 64
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters(), lr=learning_rate)
```
4. 网络训练
网络训练的过程包括多次前向传播、计算损失、反向传播和权重更新。在PyTorch中,可以通过`torch.utils.data.DataLoader`和`torch.utils.data.Dataset`来构建数据集和数据加载器。具体而言,我们需要将数据集划分为训练集和测试集,并将其转换为`Tensor`类型。接下来,我们需要循环多次训练集,并对每个小批次进行反向传播和权重更新。最后,我们可以在测试集上评估网络的性能。
```
# 划分训练集和测试集
train_set = datasets.MNIST('./data', train=True, download=True, transform=transforms.ToTensor())
test_set = datasets.MNIST('./data', train=False, download=True, transform=transforms.ToTensor())
# 创建数据加载器
train_loader = torch.utils.data.DataLoader(train_set, batch_size=batch_size, shuffle=True)
test_loader = torch.utils.data.DataLoader(test_set, batch_size=batch_size, shuffle=False)
# 训练网络
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
# 前向传播
outputs = net(images)
loss = criterion(outputs, labels)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 每训练100个批次,输出一次损失和准确率
if (i+1) % 100 == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch+1, num_epochs, i+1, len(train_loader), loss.item()))
# 在测试集上评估网络
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Test Accuracy of the model on the 10000 test images: {} %'.format(100 * correct / total))
```
5. 性能测试
在训练完成后,我们可以在测试集上评估网络的性能。具体而言,我们可以计算出网络在测试集上的准确率。
```
# 在测试集上评估网络
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Test Accuracy of the model on the 10000 test images: {} %'.format(100 * correct / total))
```
以上就是利用PyTorch平台实现数字图像识别任务的主要步骤。
阅读全文
相关推荐
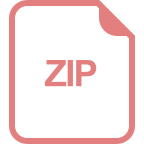
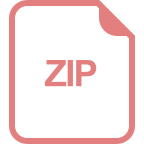




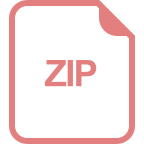
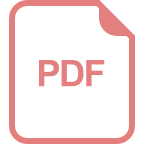
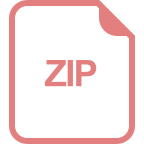
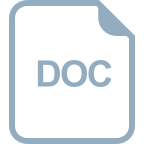
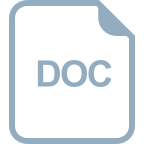
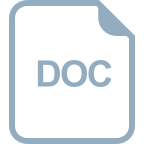
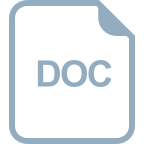
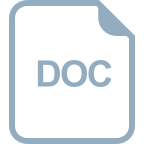
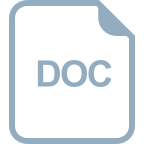
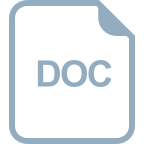
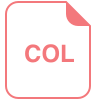
