Integer.parseInt源码
时间: 2023-11-25 19:06:53 浏览: 74
`Integer.parseInt()` 函数的具体实现如下:
```java
public static int parseInt(String s) throws NumberFormatException {
return parseInt(s,10);
}
public static int parseInt(String s, int radix)
throws NumberFormatException
{
if (s == null) {
throw new NumberFormatException("null");
}
if (radix < Character.MIN_RADIX) {
throw new NumberFormatException("radix " + radix +
" less than Character.MIN_RADIX");
}
if (radix > Character.MAX_RADIX) {
throw new NumberFormatException("radix " + radix +
" greater than Character.MAX_RADIX");
}
int result = 0;
boolean negative = false;
int i = 0, len = s.length();
int limit = -Integer.MAX_VALUE;
int multmin;
int digit;
if (len > 0) {
char firstChar = s.charAt(0);
if (firstChar < '0') { //处理负数
if (firstChar == '-') {
negative = true;
limit = Integer.MIN_VALUE;
} else if (firstChar != '+')
throw NumberFormatException.forInputString(s);
if (len == 1) // 如果只有一个符号,则抛出异常
throw NumberFormatException.forInputString(s);
i++;
}
multmin = limit / radix;
while (i < len) {
digit = Character.digit(s.charAt(i++),radix);
if (digit < 0) // 如果字符不是数字,则抛出异常
throw NumberFormatException.forInputString(s);
if (result < multmin)
throw NumberFormatException.forInputString(s);
result *= radix;
if (result < limit + digit)
throw NumberFormatException.forInputString(s);
result -= digit;
}
} else {
throw NumberFormatException.forInputString(s);
}
return negative ? result : -result;
}
```
在实现中,`parseInt()` 函数会先将传入的字符串转换为整数,如果转换失败则会抛出 `NumberFormatException` 异常。它的具体实现过程如下:
1. 首先判断传入的参数是否为 `null`,如果是则抛出异常;
2. 然后判断传入的进制数是否合法,如果不在 `2` 到 `36` 的范围内则抛出异常;
3. 接着判断字符串的第一个字符,如果是 `-` 则表示是负数,否则如果是 `+` 则忽略;
4. 如果字符串只有一个符号则抛出异常;
5. 从字符串的第一个有效数字开始,按照进制数将每个字符转换为数字,然后计算出整数;
6. 如果字符串中包含非数字字符,则抛出异常;
7. 如果整数超出了 `int` 类型的范围,则抛出异常;
8. 如果字符串的长度为 `0`,则抛出异常;
9. 最后将整数返回。
需要注意的是,在实现中,`parseInt()` 函数会使用 `Character.digit()` 函数将字符转换为数字。如果字符不是数字,则返回 `-1`。如果传入的字符串包含非数字字符,则会在第一次调用 `digit()` 函数时抛出异常。
阅读全文
相关推荐
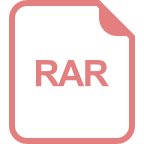

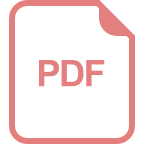
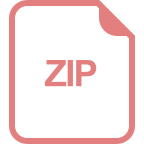
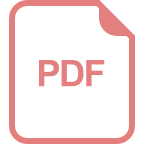
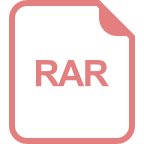
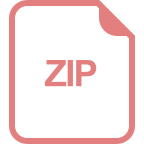
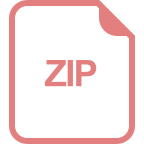
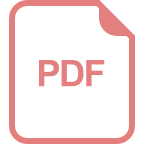
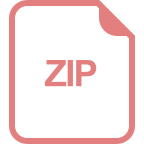
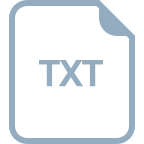
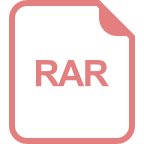
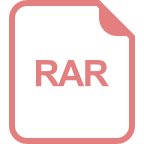
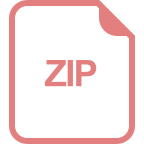
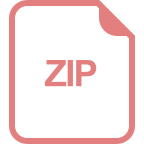
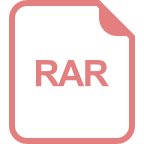
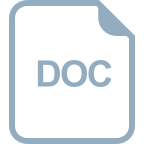