C 语言 编写学生基本信息管理程序。用链表存储一个班的学生信息(最多100位学生),每个学生的信息有:学号,姓名,性别,联系电话和家庭住址。需要实现的功能有:新建学生信息链表,对学生信息进行增加、删除和查找。有菜单显示,全部代码
时间: 2024-09-17 16:01:56 浏览: 27
在C语言中编写学生基本信息管理程序,我们可以采用链表数据结构来实现,这里是一个简单的示例,包括链表的创建、插入、删除和搜索功能:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Student {
int id;
char name[50];
char gender[10];
char phone[20];
char address[150];
struct Student* next; // 指向下一个学生的指针
} Student;
// 创建一个新的学生节点
Student* create_student(int id, char* name, char* gender, char* phone, char* address) {
Student* new_student = (Student*)malloc(sizeof(Student));
new_student->id = id;
strcpy(new_student->name, name);
strcpy(new_student->gender, gender);
strcpy(new_student->phone, phone);
strcpy(new_student->address, address);
new_student->next = NULL;
return new_student;
}
// 添加新学生到链表末尾
void add_student(Student** head, int id, char* name, char* gender, char* phone, char* address) {
if (*head == NULL) {
*head = create_student(id, name, gender, phone, address);
} else {
Student* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = create_student(id, name, gender, phone, address);
}
}
// 删除学生
void delete_student(Student** head, int id) {
if (*head == NULL) return;
if ((*head)->id == id) {
Student* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Student* current = *head;
while (current->next != NULL && current->next->id != id) {
current = current->next;
}
if (current->next != NULL) {
Student* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
// 查找学生
Student* search_student(Student* head, int id) {
Student* current = head;
while (current != NULL) {
if (current->id == id) {
return current;
}
current = current->next;
}
return NULL;
}
int main() {
Student* student_list = NULL;
int choice, id;
char name[50], gender[10], phone[20], address[150];
printf("欢迎使用学生信息管理系统\n");
while (1) {
printf("\n请选择操作:\n1. 新建学生\n2. 插入学生\n3. 删除学生\n4. 查找学生\n5. 退出\n");
scanf("%d", &choice);
switch(choice) {
case 1:
printf("请输入学生信息:\n");
scanf("%d", &id);
scanf("%s", name);
scanf("%s", gender);
scanf("%s", phone);
scanf("%s", address);
add_student(&student_list, id, name, gender, phone, address);
break;
// 其他选项的处理...
case 5:
printf("感谢使用,再见!\n");
exit(0);
default:
printf("无效选择,请重新输入。\n");
}
}
return 0;
}
```
这是一个基本的实现,实际应用中可能需要加入更多的错误检查和用户界面改进。注意,在这个例子中,我们假设所有输入都是有效的。
阅读全文
相关推荐










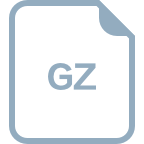




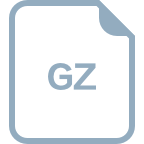
