ByteArrayOutputStream定义
时间: 2023-11-19 12:55:19 浏览: 34
ByteArrayOutputStream是一个对字节进行操作的内存操作流,它对byte类型数据进行写入,并相当于一个中间缓冲层,将数据写入到文件等其他outputStream。它继承了OutputStream类,其中包含了数据存储的地方和计数器,分别为buf和count。具体定义如下:
```java
public class ByteArrayOutputStream extends OutputStream {
// 数据存储的地方
protected byte buf[];
// 计数器,表示数据的个数
protected int count;
// 构造函数,创建一个新的ByteArrayOutputStream
public ByteArrayOutputStream() {
this(32);
}
// 构造函数,创建一个新的ByteArrayOutputStream,指定初始大小
public ByteArrayOutputStream(int size) {
if (size < 0) {
throw new IllegalArgumentException("Negative initial size: "
+ size);
}
buf = new byte[size];
}
// 将指定的字节写入此ByteArrayOutputStream
public synchronized void write(int b) {
int newcount = count + 1;
if (newcount > buf.length) {
buf = Arrays.copyOf(buf, Math.max(buf.length << 1, newcount));
}
buf[count] = (byte) b;
count = newcount;
}
// 将指定的字节数组写入此ByteArrayOutputStream
public synchronized void write(byte b[], int off, int len) {
if ((off < 0) || (off > b.length) || (len < 0) ||
((off + len) - b.length > 0)) {
throw new IndexOutOfBoundsException();
}
if (len == 0) {
return;
}
int newcount = count + len;
if (newcount > buf.length) {
buf = Arrays.copyOf(buf, Math.max(buf.length << 1, newcount));
}
System.arraycopy(b, off, buf, count, len);
count = newcount; }
// 将此ByteArrayOutputStream的缓冲区作为一个字节数组返回
public synchronized byte toByteArray()[] {
return Arrays.copyOf(buf, count);
}
// 返回此ByteArrayOutputStream的当前大小
public synchronized int size() {
return count;
}
// 将此ByteArrayOutputStream重置为零个字节的新流
public synchronized void reset() {
count = 0;
}
// 将此流的内容转换为字符串,使用平台默认字符集
public synchronized String toString() {
return new String(buf, 0, count);
}
// 将此流的内容转换为字符串,使用指定的字符集
public synchronized String toString(String charsetName)
throws UnsupportedEncodingException
{
return new String(buf, 0, count, charsetName);
}
// 将此流的内容写入到指定的输出流中
public synchronized void writeTo(OutputStream out) throws IOException {
out.write(buf, 0, count);
}
// 关闭此流,但是此方法没有任何效果
public void close() throws IOException {}
}
```
相关推荐
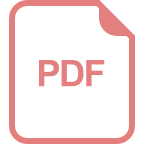
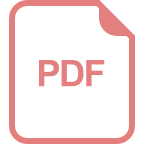
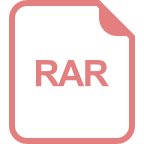














