代码解析:public static String fetchFrame(String videoPath) { FFmpegFrameGrabber ff = null; byte[] data = null; ByteArrayOutputStream os = new ByteArrayOutputStream(); try { ff = new FFmpegFrameGrabber(videoPath); ff.start(); int lenght = ff.getLengthInFrames(); int i = 0; Frame f = null; while (i < lenght) { // 过滤前5帧,避免出现全黑的图片 这个根据自己的情况来定,这里就以5秒作为测试 f = ff.grabFrame(); if ((i > 5) && (f.image != null)) { break; } i++; } BufferedImage bi = new Java2DFrameConverter().getBufferedImage(f); String rotate = ff.getVideoMetadata("rotate"); if (rotate != null) { bi = rotate(bi, Integer.parseInt(rotate)); } ImageIO.write(bi, "jpg", os); } catch (Exception e) { e.printStackTrace(); } finally { try { if (ff != null) { ff.stop(); } } catch (FrameGrabber.Exception e) { e.printStackTrace(); } } BASE64Encoder encoder = new BASE64Encoder(); return "data:image/jpg;base64,"+encoder.encode(os.toByteArray()); }
时间: 2024-02-10 13:35:16 浏览: 27
这段代码是一段Java代码,主要功能是从视频文件中提取第一帧图片并将其转换为BASE64编码的字符串。
具体的代码解析如下:
1. 导入需要的类:
```
import org.bytedeco.javacv.*;
import sun.misc.BASE64Encoder;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import javax.imageio.ImageIO;
```
2. 定义fetchFrame方法,参数为视频文件路径videoPath,返回值为BASE64编码的字符串。
```
public static String fetchFrame(String videoPath) {
```
3. 初始化一些变量,包括FFmpegFrameGrabber对象ff,字节数组data,输出流ByteArrayOutputStream对象os等。
```
FFmpegFrameGrabber ff = null;
byte[] data = null;
ByteArrayOutputStream os = new ByteArrayOutputStream();
```
4. 使用FFmpegFrameGrabber对象ff从视频文件videoPath中获取第一帧图片,并将其转换为BufferedImage对象bi。
```
ff = new FFmpegFrameGrabber(videoPath);
ff.start();
int lenght = ff.getLengthInFrames();
int i = 0;
Frame f = null;
while (i < lenght) {
f = ff.grabFrame();
if ((i > 5) && (f.image != null)) {
break;
}
i++;
}
BufferedImage bi = new Java2DFrameConverter().getBufferedImage(f);
```
5. 判断视频是否旋转,如果旋转则进行旋转操作。
```
String rotate = ff.getVideoMetadata("rotate");
if (rotate != null) {
bi = rotate(bi, Integer.parseInt(rotate));
}
```
6. 将BufferedImage对象bi转换为jpg格式的图片,并将其写入ByteArrayOutputStream对象os中。
```
ImageIO.write(bi, "jpg", os);
```
7. 将ByteArrayOutputStream对象os中的数据进行BASE64编码,并返回BASE64编码的字符串。
```
BASE64Encoder encoder = new BASE64Encoder();
return "data:image/jpg;base64,"+encoder.encode(os.toByteArray());
```
至此,这段Java代码的功能就是从视频文件中提取第一帧图片并将其转换为BASE64编码的字符串。
相关推荐
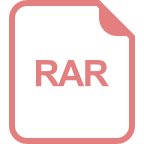
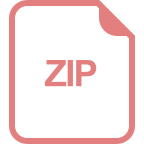
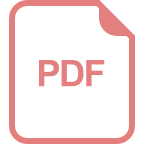














