DESKTOP-TS windows操作系統中可以复制文件夹,比如把DAVfromllday11文件夹,复制到EAItolday11文件夹下。但是ava没有提供直接复制文件夹的方法,请编写程序从健品接收两个文件夹路径把其中一个文件夹中(包含内客持贝到另一个文件夹中
时间: 2023-07-29 17:10:28 浏览: 50
你可以使用Java中的文件操作类来实现复制文件夹的功能。以下是一个示例程序:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.StandardCopyOption;
public class FolderCopy {
public static void main(String[] args) {
// 从命令行参数获取需要复制的文件夹路径和目标文件夹路径
if (args.length != 2) {
System.out.println("Usage: java FolderCopy <source folder> <destination folder>");
return;
}
String sourceFolder = args[0];
String destFolder = args[1];
// 创建目标文件夹
File destDir = new File(destFolder);
destDir.mkdirs();
// 遍历源文件夹下的所有文件和子文件夹,递归复制
File sourceDir = new File(sourceFolder);
File[] files = sourceDir.listFiles();
for (File file : files) {
String fileName = file.getName();
File dest = new File(destDir, fileName);
if (file.isDirectory()) {
try {
copyFolder(file.toPath(), dest.toPath());
} catch (IOException e) {
System.out.println("Error copying folder " + file.getPath() + ": " + e.getMessage());
}
} else {
try {
Files.copy(file.toPath(), dest.toPath(), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
System.out.println("Error copying file " + file.getPath() + ": " + e.getMessage());
}
}
}
System.out.println("Folder copy completed.");
}
// 复制文件夹的递归方法
private static void copyFolder(java.nio.file.Path source, java.nio.file.Path dest) throws IOException {
Files.walk(source).forEach(file -> {
try {
Files.copy(file, dest.resolve(source.relativize(file)), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
System.out.println("Error copying file " + file.toString() + ": " + e.getMessage());
}
});
}
}
```
在命令行中运行该程序,输入源文件夹路径和目标文件夹路径,即可完成文件夹复制。例如:
```
java FolderCopy C:\DAVfromllday11 C:\EAItolday11
```
这将把 `C:\DAVfromllday11` 文件夹中的所有文件和子文件夹复制到 `C:\EAItolday11` 文件夹中。
相关推荐
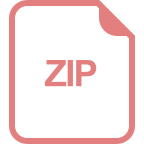
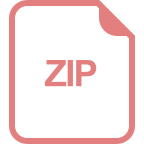














