用c++写以班级为单位进行选题,题目信息以文件形式存放, 包括:编号、题目、任务要求、完成时间 功能要求: 1学生信息包括:学号、姓名、班级; 2输入学号,随机抽取题目,限定每个题目只能被一个学生选中 3选题结果以文件形式存放,可以随时进行查询操作
时间: 2024-02-06 21:02:09 浏览: 35
好的,我可以为您提供一个简单的 C++ 实现,实现班级选题的功能。以下是代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
// 学生信息结构体
struct Student {
string id; // 学号
string name; // 姓名
string cls; // 班级
};
// 题目信息结构体
struct Topic {
int id; // 题目编号
string title; // 题目名称
string desc; // 任务要求
string time; // 完成时间
bool taken; // 是否已被选中
};
// 学生选题信息结构体
struct StudentTopic {
Student student; // 学生信息
int topicId; // 选中的题目编号
};
// 读取学生信息文件
vector<Student> readStudentFile(string filename) {
vector<Student> students;
ifstream input(filename);
string id, name, cls;
while (input >> id >> name >> cls) {
students.push_back({id, name, cls});
}
input.close();
return students;
}
// 读取题目信息文件
vector<Topic> readTopicFile(string filename) {
vector<Topic> topics;
ifstream input(filename);
int id;
string title, desc, time;
while (input >> id >> title >> desc >> time) {
topics.push_back({id, title, desc, time, false});
}
input.close();
return topics;
}
// 保存学生选题信息
void saveStudentTopic(string filename, StudentTopic st) {
ofstream output(filename, ios::app);
output << st.student.id << " " << st.student.name << " " << st.student.cls << " " << st.topicId << endl;
output.close();
}
// 查找学生选题信息
StudentTopic findStudentTopic(string filename, string id) {
ifstream input(filename);
string sid, name, cls;
int topicId;
while (input >> sid >> name >> cls >> topicId) {
if (sid == id) {
input.close();
return { {sid, name, cls}, topicId };
}
}
input.close();
return { {"", "", ""}, 0 };
}
int main() {
// 读取学生信息和题目信息文件
vector<Student> students = readStudentFile("students.txt");
vector<Topic> topics = readTopicFile("topics.txt");
// 随机抽取题目并选题
srand(time(NULL));
string studentId;
cout << "请输入学生学号:";
cin >> studentId;
int topicIndex = rand() % topics.size();
while (topics[topicIndex].taken) {
topicIndex = rand() % topics.size();
}
topics[topicIndex].taken = true;
StudentTopic st = { { "", "", "" }, 0 };
for (auto s : students) {
if (s.id == studentId) {
st = { s, topics[topicIndex].id };
break;
}
}
if (st.student.id == "") {
cout << "未找到该学生" << endl;
return 1;
}
// 保存学生选题信息
saveStudentTopic("student_topics.txt", st);
// 输出选题信息
cout << "选题成功:" << st.student.name << " 同学,题目编号为 " << st.topicId << endl;
// 查询学生选题信息
StudentTopic stFound = findStudentTopic("student_topics.txt", studentId);
if (stFound.student.id == "") {
cout << "未找到该学生选题信息" << endl;
} else {
cout << "该学生已选题,选题信息为:" << stFound.student.name << " 同学,题目编号为 " << stFound.topicId << endl;
}
return 0;
}
```
在这个实现中,我们使用了三个结构体来存储学生信息、题目信息和学生选题信息。首先,通过 `readStudentFile` 和 `readTopicFile` 函数,我们分别读取了学生信息和题目信息文件,并将读取到的信息存储到相应的结构体中。接下来,我们使用 `rand` 函数来随机抽取一个未被选中的题目,并将其与指定学生关联。最后,使用 `saveStudentTopic` 函数将学生选题信息保存到文件中,并使用 `findStudentTopic` 函数实现了查询学生选题信息的功能。
当然,这只是一个简单的实现,您可以根据实际需求进行修改和扩展。同时,该实现也存在一些问题,例如没有对文件读取、写入过程中的错误进行处理等等,需要根据实际情况进行完善。
相关推荐
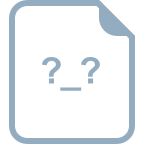











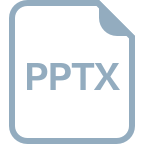
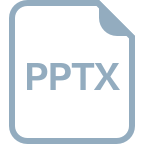