帮我完成文件里的第二道题和第三道题,用C++完成
时间: 2024-10-20 10:12:21 浏览: 30
好的,我来帮助您完成“最快路线问题”和“汽车加油问题”的 C++ 实现。
### 最快路线问题
#### 问题描述
某市民开车想从 A 点至 H 点,需要找到最快的路线。给定每条路的路程和速度,其中部分路段因事故导致速度降低。
#### 算法思路
1. 将每条路线的路程和速度转换为通过时间。
2. 使用 Dijkstra 算法求解单源最短路径问题。
#### 代码实现
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
using namespace std;
const int INF = numeric_limits<int>::max();
struct Edge {
int to;
double cost;
};
void dijkstra(const vector<vector<Edge>>& graph, int start, vector<double>& dist) {
priority_queue<pair<double, int>, vector<pair<double, int>>, greater<>> pq;
dist[start] = 0;
pq.push({0, start});
while (!pq.empty()) {
auto [d, u] = pq.top();
pq.pop();
if (d > dist[u]) continue;
for (const auto& edge : graph[u]) {
int v = edge.to;
double weight = edge.cost;
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.push({dist[v], v});
}
}
}
}
int main() {
int n = 8;
vector<vector<Edge>> graph(n);
// 建图
graph[0].push_back({1, 9.0 / 40}); // A -> B
graph[0].push_back({2, 7.0 / 50}); // A -> C
graph[0].push_back({3, 9.0 / 80}); // A -> D
graph[1].push_back({2, 6.0 / 50}); // B -> C
graph[1].push_back({4, 12.0 / 50}); // B -> E
graph[1].push_back({5, 12.0 / 40}); // B -> F
graph[2].push_back({3, 8.0 / 50}); // C -> D
graph[2].push_back({4, 9.0 / 50}); // C -> E
graph[3].push_back({4, 10.0 / 50}); // D -> E
graph[3].push_back({6, 10.0 / 30}); // D -> G
graph[4].push_back({5, 7.0 / 50}); // E -> F
graph[4].push_back({6, 8.0 / 50}); // E -> G
graph[4].push_back({7, 7.0 / 20}); // E -> H
graph[5].push_back({7, 9.0 / 80}); // F -> H
graph[6].push_back({7, 11.0 / 80}); // G -> H
vector<double> dist(n, INF);
dijkstra(graph, 0, dist); // 从 A 点开始
cout << "从 A 到 H 的最短时间为: " << dist[7] << "小时" << endl;
return 0;
}
```
### 汽车加油问题
#### 问题描述
一辆汽车加满油后可以行驶 N 公里,汽车从起点 A 开往终点 B,途中有多座加油站。目标是在最少的加油次数内到达终点。
#### 算法思路
使用贪心算法,每次尽可能远地行驶,直到无法继续行驶时在最近的加油站加油。
#### 代码实现
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int minRefills(vector<int>& stops, int tank, int destination) {
stops.insert(stops.begin(), 0); // 添加起点
stops.push_back(destination); // 添加终点
int numRefills = 0;
int currentRefill = 0;
while (currentRefill <= stops.size() - 2) {
int lastRefill = currentRefill;
while (currentRefill <= stops.size() - 2 && stops[currentRefill + 1] - stops[lastRefill] <= tank) {
currentRefill++;
}
if (currentRefill == lastRefill) {
return -1; // 无法到达终点
}
if (currentRefill < stops.size() - 1) {
numRefills++;
}
}
return numRefills;
}
int main() {
int N = 600;
vector<int> stops = {300, 500, 700, 1100, 1400, 1600, 1900, 2200};
int destination = 2300;
int result = minRefills(stops, N, destination);
if (result != -1) {
cout << "最少加油次数: " << result << endl;
} else {
cout << "无法到达终点" << endl;
}
return 0;
}
```
以上是两道题目的完整实现。希望对您有所帮助!如果有任何问题或需要进一步解释,请随时告诉我。
阅读全文
相关推荐



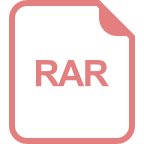

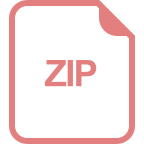

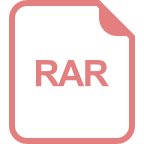
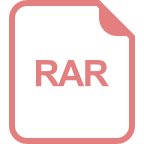



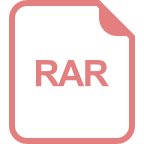
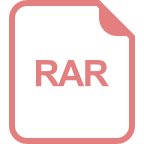




