设计一个算法,计算一个仅包含字母字符的顺序串s中的最大字母出现的次数,使用c++语言
时间: 2024-10-18 08:13:04 浏览: 47
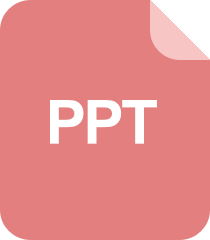
将若干字符串按字母顺序由小到大输出。-C++程序设计(谭浩强完整版)
要设计一个C++算法来计算一个只包含字母字符的字符串`s`中每个字母的最大出现次数,我们可以使用哈希表(unordered_map)。以下是步骤:
1. 定义一个哈希表存储每个字母及其出现次数。
2. 遍历字符串`s`中的每一个字符。
3. 如果字符不在哈希表中,添加它并初始化计数为1。
4. 如果字符已经在哈希表中,增加其计数。
5. 比较当前字母的计数与之前遇到的最大值,并更新最大值。
6. 最终返回每个字母的最大出现次数。
下面是相应的C++代码实现:
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
std::unordered_map<char, int> maxCount(const std::string& s) {
std::unordered_map<char, int> counts;
for (char c : s) {
if (isalpha(c)) { // 检查是否为字母
counts[c]++;
counts[c] = std::max(counts[c], counts.count(c) ? counts[c] : 1); // 更新最大值
}
}
return counts;
}
int main() {
std::string str;
std::cout << "Enter a string containing only alphabetical characters: ";
std::cin >> str;
std::unordered_map<char, int> result = maxCount(str);
for (const auto &pair : result) {
char letter = pair.first;
int count = pair.second;
std::cout << "Character '" << letter << "' appears " << count << " times.\n";
}
return 0;
}
```
阅读全文
相关推荐



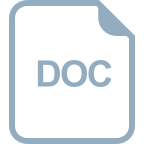
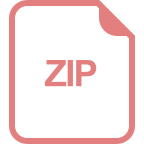
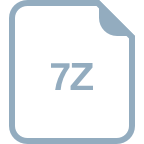
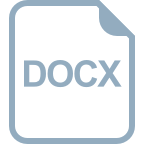
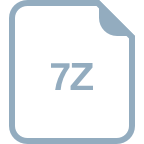
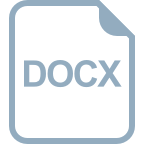
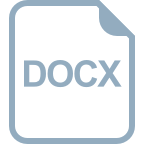