编写一个程序,能够接收两组以'小时:分钟:秒'格式表示的时间输入,然后计算并输出这两段时间之间的间隔(以相同格式显示)。
时间: 2024-11-04 09:11:24 浏览: 14
为了编写一个C语言程序来计算两个时间之间的时间差,你可以使用`struct`来定义时间结构体,并用`time_t`类型处理实际的时长。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义时间结构体
struct Time {
int hours;
int minutes;
int seconds;
};
// 函数来从字符串转换为时间结构体
struct Time stringToTime(const char *str) {
struct tm tm = {};
sscanf(str, "%d:%d:%d", &tm.tm_hour, &tm.tm_min, &tm.tm_sec);
return (struct Time) { tm.tm_hour, tm.tm_min, tm.tm_sec };
}
// 计算两个时间之间的差
void calculateDifference(struct Time t1, struct Time t2, struct Time* difference) {
time_t diff = mktime(&(*difference).hours + &t2.hours,
&(*difference).minutes + &t2.minutes,
&(*difference).seconds + &t2.seconds,
tm_year, tm_mon, tm_mday, tm_hour, tm_min, tm_sec, tm_wday, tm_yday, tm_isdst);
// 如果diff是负数,说明t1在t2之后,取其补码
if (diff < 0) {
diff = -diff;
}
// 将时间戳转换回'小时:分钟:秒'格式
char buffer[64];
strftime(buffer, sizeof(buffer), "%H:%M:%S", localtime(&diff));
difference->hours = atoi(buffer + 2); // 提取小时
difference->minutes = atoi(buffer + 5); // 提取分钟
difference->seconds = atoi(buffer + 8); // 提取秒
}
int main() {
char str1[100], str2[100];
struct Time time1, time2, difference;
printf("Enter first time (format: HH:MM:SS): ");
fgets(str1, sizeof(str1), stdin);
str1[strlen(str1)-1] = '\0'; // remove newline
printf("Enter second time (format: HH:MM:SS): ");
fgets(str2, sizeof(str2), stdin);
str2[strlen(str2)-1] = '\0';
time1 = stringToTime(str1);
time2 = stringToTime(str2);
calculateDifference(time1, time2, &difference);
printf("The difference between the two times is: %02d:%02d:%02d\n", difference.hours, difference.minutes, difference.seconds);
return 0;
}
```
在这个程序中,我们首先定义了一个`struct Time`来存储小时、分钟和秒,然后创建了`stringToTime`函数用于解析字符串到时间结构体。`calculateDifference`函数计算两个时间之间的差值,如果时间顺序反了,就取负数。最后,在`main`函数中获取用户输入,调用这两个函数并打印结果。
阅读全文
相关推荐
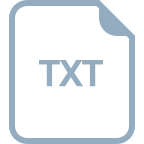
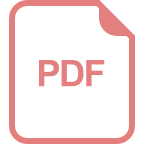
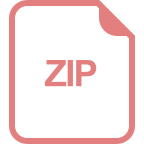















