面向对象Python图的遍历代码
时间: 2024-06-05 16:04:32 浏览: 12
在Python中,我们可以使用类来表示图,常用的遍历算法有深度优先搜索(DFS)和广度优先搜索(BFS)。以下是一个简单的示例代码,使用DFS遍历图:
```
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def DFS_util(self, v, visited):
visited.add(v)
print(v, end=' ')
for neighbour in self.graph[v]:
if neighbour not in visited:
self.DFS_util(neighbour, visited)
def DFS(self, start):
visited = set()
self.DFS_util(start, visited)
```
这里我们使用了Python中的`defaultdict`来表示邻接表,其中`add_edge`方法用于添加边。`DFS_util`方法实现了递归的深度优先遍历,其中`visited`用于记录已经遍历过的节点,`print`语句用于输出遍历结果。`DFS`方法则是对外的接口方法,接收一个起点作为参数。
如果你想使用BFS算法遍历图,则可以使用Python内置的队列数据结构来实现。以下是一个简单的示例代码:
```
from collections import deque
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def BFS(self, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
v = queue.popleft()
print(v, end=' ')
for neighbour in self.graph[v]:
if neighbour not in visited:
visited.add(neighbour)
queue.append(neighbour)
```
这里我们使用了Python内置的双端队列`deque`来作为BFS算法中的队列数据结构。`BFS`方法使用了迭代的方式进行广度优先遍历,同样也是接收一个起点作为参数。
相关推荐
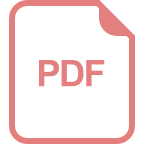
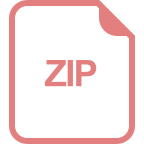
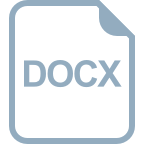
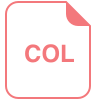
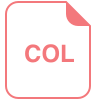
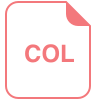
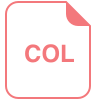
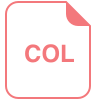









