python scores = {"Michael":80,"Ethan":55,"Joshua":67,"Alexander":99,"James":74,"Mark":84,"Tom":49,"Henry":88} 要求: 1. 遍历学生成绩的字典,计算的全班的平均值
时间: 2024-01-02 07:02:22 浏览: 32
要计算全班的平均值,可以使用以下代码遍历学生成绩的字典,并计算平均值:
```python
scores = {"Michael":80,"Ethan":55,"Joshua":67,"Alexander":99,"James":74,"Mark":84,"Tom":49,"Henry":88}
total_score = 0
num_students = len(scores)
for score in scores.values():
total_score += score
average_score = total_score / num_students
print("全班的平均值为:", average_score)
```
相关问题
file:/D:/python/scores/race.txt
好的,我已经下载了文本数据。我将为你编写程序,统计敏感词的词频,并将结果按照词频从小到大排序。以下是代码实现:
```python
import jieba
import re
from collections import Counter
# 读取敏感词文件
with open('CDIAL-BIAS-race.txt', 'r', encoding='utf-8') as f:
sensitive_words = [line.strip() for line in f]
# 读取文本数据
with open('race.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用结巴分词对文本进行分词
words = jieba.cut(text)
# 统计每个敏感词的词频
word_counts = Counter()
for word in words:
if word in sensitive_words:
word_counts[word] += 1
# 按照词频从小到大排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1])
# 输出结果
for word, count in sorted_word_counts:
print(f'{word}: {count}')
```
请将代码保存为.py文件并运行,程序将输出每个敏感词的词频,按照词频从小到大排序。
np.argsort(scores, axis=0)[::-1, 0]
这是一个 Python 中的 NumPy 数组方法,它返回的是数组中元素从小到大排列后的下标索引值。具体实现方法是,将 scores 数组沿着第0维进行排序,然后将排序结果进行反转,最后返回排序后的索引值数组。如果需要以降序形式返回排序结果,则还需要在下标数组前面加上一个负号。
相关推荐
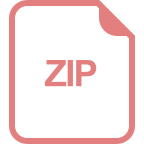
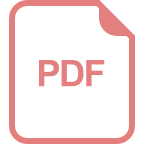
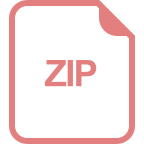












