对上述问题具体的C语言代码
时间: 2024-10-24 07:18:41 浏览: 3
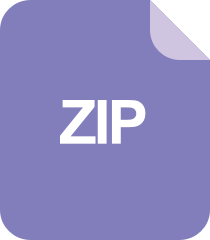
兔子繁衍问题-C语言代码
为了编写一个C语言函数来解决这个问题,我们需要采用递归的方式来恢复二叉树。以下是简化的C语言代码片段,用于计算二叉树的高度:
```c
#include <stdio.h>
#include <string.h>
// 定义二叉树节点结构
typedef struct TreeNode {
char data;
struct TreeNode *left, *right;
} TreeNode;
// 将字符数组转换成二叉树节点
TreeNode* buildTree(char pre[], char in[]) {
int i = 0, j = 0;
// 找到当前节点的元素在中序遍历中的位置
for (i = 0; in[j] != '\0'; ++j)
if (in[j] == pre[0])
break;
// 创建新节点
TreeNode *root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = pre[0];
root->left = buildTree(pre + 1, in);
root->right = buildTree(pre + i + 1, in + j + 1);
return root;
}
// 计算二叉树的高度
int height(TreeNode* node) {
if (node == NULL)
return 0;
else
return 1 + MAX(height(node->left), height(node->right));
}
// 主函数
int main() {
int N;
scanf("%d", &N);
char pre[N+1], in[N+1]; // 假设输入的字符串已经存入这里
scanf(" %s %s", pre, in);
TreeNode* root = buildTree(pre, in);
printf("The height of the binary tree is: %d\n", height(root));
free(root); // 释放内存
return 0;
}
```
在这个代码中,`buildTree` 函数用于根据先序和中序遍历构造二叉树,`height` 函数则递归地计算树的高度。在`main`函数中,我们读取输入、创建树并打印结果。
阅读全文
相关推荐
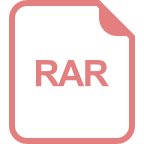
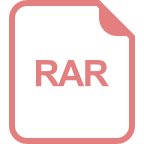
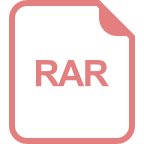
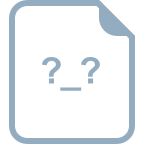
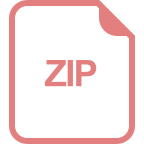
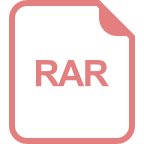
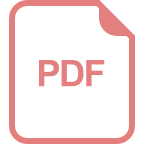
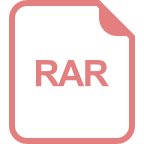
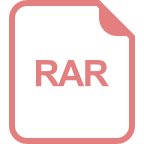
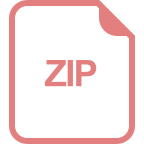
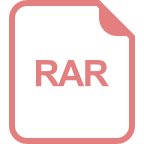
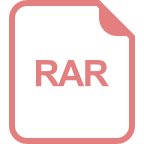
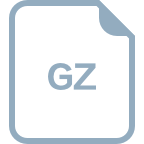
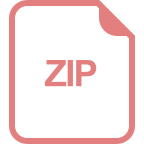
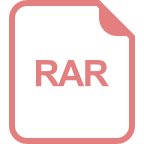
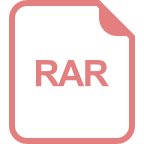
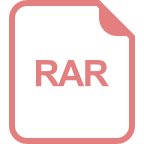
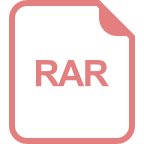