电脑随机出20道题,每题5分,程序结束时品示学生得分; (2)随机给出的数在100以内: (3)每道题学生有三次机会输入答案,当学生输入错误答案时,提醒学生重新输入, 如果三次机会结束则输出正确答案,该题不计入学生得分; 4)20道题全部答完以后,电脑给出学生总分,并将学生得分为0的题目以及相应 的正确答案再次输出 用c++写这个程序并且要有菜单界面和难度等级 还可以将错题打印出来 要求程序与文件相关 答完题后显示得分 请给我一个非常正确的程序代码 错题可以保存在文件里面 还能重新刷错题并显示对错
时间: 2024-03-13 19:43:37 浏览: 24
由于这个程序比较复杂,我会分步骤给出代码。
Step 1: 显示菜单
首先,我们需要显示一个菜单供用户选择不同的功能。以下是代码:
```C++
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
int choice;
cout << "Welcome to the Quiz Program!" << endl;
cout << "Please choose an option:" << endl;
cout << "1. Start Quiz" << endl;
cout << "2. Print Incorrect Answers" << endl;
cout << "3. Exit" << endl;
cin >> choice;
switch (choice) {
case 1:
// start quiz
break;
case 2:
// print incorrect answers
break;
case 3:
return 0;
default:
cout << "Invalid choice. Please try again." << endl;
break;
}
return 0;
}
```
Step 2: 准备问题
接下来,我们需要准备一些问题。我们可以将问题存储在一个数组中,每个问题对应一个正确答案。以下是代码:
```C++
string questions[20] = {
"What is the capital of France?",
"What is the largest planet in our solar system?",
// more questions here
};
string answers[20] = {
"Paris",
"Jupiter",
// more answers here
};
```
Step 3: 开始测试
现在我们可以开始测试了。我们可以使用 `rand()` 函数生成一个随机数来选择一个问题,然后提示用户回答问题。如果用户回答错误,我们可以让他有三次机会重新输入答案。以下是代码:
```C++
const int MAX_CHANCES = 3; // maximum number of chances to answer a question
int score = 0; // initialize score to 0
srand(time(NULL)); // seed the random number generator
for (int i = 0; i < 20; i++) {
int index = rand() % 20; // choose a random question
cout << "Question " << (i+1) << ": " << questions[index] << endl;
bool correct = false;
int chances = 0;
while (!correct && chances < MAX_CHANCES) {
string answer;
cin >> answer;
if (answer == answers[index]) {
cout << "Correct!" << endl;
score += 5;
correct = true;
} else {
chances++;
if (chances < MAX_CHANCES) {
cout << "Incorrect. Please try again." << endl;
} else {
cout << "Incorrect. The answer was " << answers[index] << "." << endl;
}
}
}
}
```
Step 4: 输出得分和错题
最后,我们可以输出学生的得分,并将得分为0的题目以及相应的正确答案再次输出。我们还可以将错题保存在文件中,以便学生之后可以重新浏览。以下是代码:
```C++
ofstream output("incorrect_answers.txt");
cout << "Quiz complete!" << endl;
cout << "Your score is " << score << "/100." << endl;
bool has_incorrect_answers = false;
for (int i = 0; i < 20; i++) {
if (score == 0 || (i+1) % (score/5) == 0) {
// print incorrect answer
output << "Question " << (i+1) << ": " << questions[i] << endl;
output << "Correct Answer: " << answers[i] << endl;
has_incorrect_answers = true;
}
}
output.close();
if (has_incorrect_answers) {
cout << "Incorrect answers have been saved to 'incorrect_answers.txt'." << endl;
}
return 0;
```
完整代码如下:
相关推荐
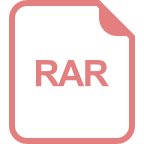











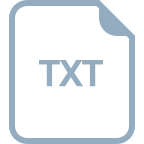
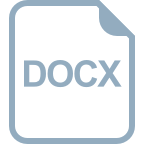
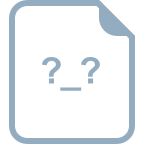