netty实现即时通讯
时间: 2023-09-13 14:10:44 浏览: 129
Netty是一款基于NIO的网络通讯框架,它提供了高性能、高可靠性的网络编程能力,因此可以用来实现即时通讯。以下是一个简单的使用Netty实现即时通讯的示例:
1. 创建一个Netty的Server端和Client端
```java
// Server端
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定端口,开始接收进来的连接
ChannelFuture f = b.bind(port).sync();
// 等待服务器 socket 关闭 。
// 在这个例子中,这不会发生,但你可以优雅地关闭你的服务器。
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
// Client端
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ClientHandler());
}
});
// 连接服务端
ChannelFuture f = b.connect(host, port).sync();
// 等待连接关闭
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
2. 编写ServerHandler和ClientHandler
```java
// ServerHandler
public class ServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理客户端发送过来的消息
ByteBuf in = (ByteBuf) msg;
try {
System.out.println("Server received: " + in.toString(CharsetUtil.UTF_8));
// 将消息返回给客户端
ctx.write(in);
ctx.flush();
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 当出现异常时关闭连接
cause.printStackTrace();
ctx.close();
}
}
// ClientHandler
public class ClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) {
// 发送消息给服务端
ctx.writeAndFlush(Unpooled.copiedBuffer("Hello Server", CharsetUtil.UTF_8));
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理服务端返回的消息
ByteBuf in = (ByteBuf) msg;
try {
System.out.println("Client received: " + in.toString(CharsetUtil.UTF_8));
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 当出现异常时关闭连接
cause.printStackTrace();
ctx.close();
}
}
```
这样,我们就可以用Netty实现简单的即时通讯了。当然,实际应用中还需要考虑更多的因素,例如协议的定义和解析、消息的组装和拆解等。
阅读全文
相关推荐
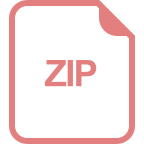
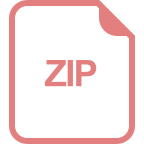
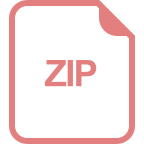
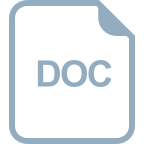


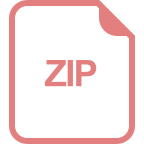
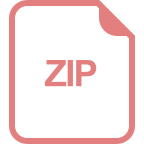
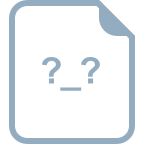
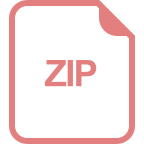
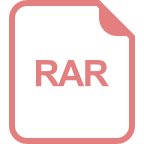
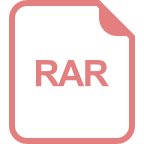
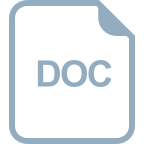
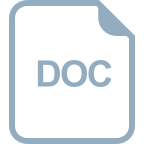
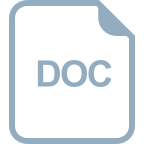
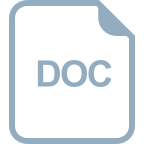
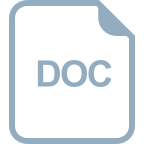
