用java自己写一个demo,接收http请求并响应,返回的响应用json格式
时间: 2023-05-23 16:04:04 浏览: 140
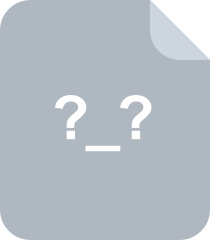
一个json格式的demo
Sure, here's a sample Java code that can receive an HTTP request and respond with a JSON-encoded response:
```
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetSocketAddress;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import org.json.JSONException;
import org.json.JSONObject;
public class DemoServer {
public static void main(String[] args) throws Exception {
HttpServer server = HttpServer.create(new InetSocketAddress(8080), 0);
server.createContext("/demo", new MyHandler());
server.setExecutor(null); // creates a default executor
server.start();
}
static class MyHandler implements HttpHandler {
@Override
public void handle(HttpExchange t) throws IOException {
String response = "";
int status = 200;
try {
// Process request
String query = t.getRequestURI().getQuery();
if (query != null && !query.isEmpty()) {
// Parse query parameters
String[] params = query.split("&");
int value = 0;
for (String param : params) {
String[] keyValue = param.split("=");
if (keyValue[0].equals("value")) {
value = Integer.parseInt(keyValue[1]);
}
}
// Generate response
JSONObject json = new JSONObject();
json.put("result", value * 2);
response = json.toString();
}
} catch (JSONException e) {
e.printStackTrace();
response = "Unexpected error";
status = 500;
}
// Send response
t.getResponseHeaders().add("Content-Type", "application/json");
t.sendResponseHeaders(status, response.length());
OutputStream os = t.getResponseBody();
os.write(response.getBytes());
os.close();
}
}
}
```
This code sets up an HTTP server that listens to port 8080 and has one endpoint "/demo". When a GET request is made to this endpoint with a query parameter named "value", the server responds with a JSON-encoded message that has a "result" field with the double of the value passed in the query. For example, a request to "http://localhost:8080/demo?value=3" will result in a response of '{"result": 6}'.
阅读全文
相关推荐
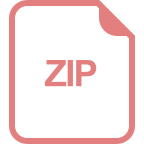


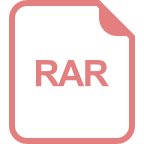
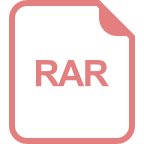
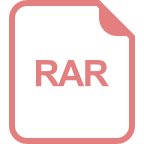
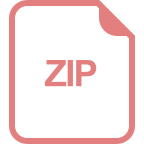
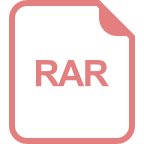
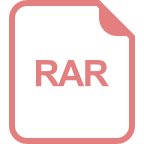
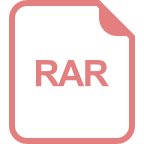
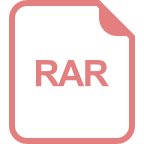
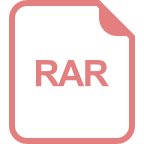
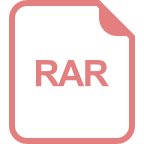
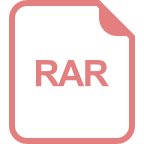
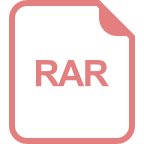
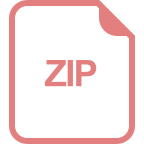
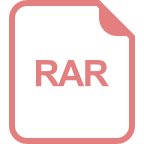