解释下 def forward(self, x, feat): z = torch.zeros_like(x) log_det = torch.zeros(z.shape[0]).to(x.device) out = torch.cat([feat, x],1) out = F.linear(out, self.first_weight*self.first_mask, self.first_bias) out = F.leaky_relu(out, negative_slope=0.2) out = self.first_ln(out) for h in range(self.hidden_layer): out = F.linear(out, self.__getattr__('middle_weight'+str(h))*self.middle_mask, self.__getattr__('middle_bias'+str(h))) out = F.leaky_relu(out, negative_slope=0.2) out = self.middle_ln[h](out) out = F.linear(out, self.last_weight*self.last_mask, self.last_bias) out = out.reshape(x.size(0), self.dim, 3*self.K-1) W, H, D = torch.chunk(out, 3, -1) z, log_det = unconstrained_RQS(x, W, H, D) return z, log_det.sum(-1)
时间: 2023-04-04 19:00:39 浏览: 67
这是一个神经网络的前向传播函数,其中 x 是输入的数据,feat 是特征向量。函数中使用了多个线性层和激活函数,其中包括 leaky_relu 和 LayerNorm。在神经网络的中间层中,使用了循环来进行多次线性变换和激活函数操作。最后,将输出的结果进行了一些处理,包括 reshape 和 chunk 操作,然后使用 unconstrained_RQS 函数进行变换,得到最终的输出 z 和 log_det。
相关问题
def predict(im0s): # 进行推理 img = torch.zeros((1, 3, imgsz, imgsz), device=device) # 初始化img _ = model(img.half() if half else img) if device.type != 'cpu' else None # 运行一次模型 # 设置数据加载器并进行推理 img = letterbox(im0s, new_shape=imgsz)[0] # 对输入图像进行resize img = img[:, :, ::-1].transpose(2, 0, 1) # BGR转RGB, 3x416x416 img = np.ascontiguousarray(img) # 返回具有相同数据和顺序的相同形状数组 img = torch.from_numpy(img).to(device) # 将numpy数组转换为张量并传递到设备上 img = img.half() if half else img.float() # 数据类型转换为float16或float32 img /= 255.0 # 将像素值从0-255映射到0.0-1.0 if img.ndimension() == 3: img = img.unsqueeze(0) # 给张量添加一个额外的纬度,输出新的张量 # 进行推理 pred = model(img)[0] # 应用非极大值抑制 pred = non_max_suppression(pred, opt_conf_thres, opt_iou_thres) # 处理检测结果 ret = [] for i, det in enumerate(pred): # 每张图片有多个检测结果 if len(det): # 将检测框位置从img_size调整到原始图像大小 det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0s.shape).round() # 输出结果 for *xyxy, conf, cls in reversed(det): label = f'{names[int(cls)]}' # 输出结果的标签信息 prob = round(float(conf) * 100, 2) # 置信度转换 ret_i = [label, prob, xyxy] # 将结果存入list ret.append(ret_i) # 返回信息:标签信息 'face' 'smoke' 'drink' 'phone',对应的置信度和位置信息(检测框) return ret
这段代码看起来像是对一个目标检测模型进行推理的代码。具体来说,输入一张图片,经过一系列处理(包括resize、数据类型转换等),然后通过模型得到预测结果。这些预测结果经过非极大值抑制后,再进行位置调整和输出结果解析,最终返回一个包含标签信息、置信度和位置信息的列表。其中标签信息包括了人脸、吸烟、饮酒和使用手机等。
yolov7train.py详解
yolov7train.py 是使用 YOLOv7 算法进行目标检测的训练脚本。下面对 yolov7train.py 的主要代码进行简单的解释:
1. 导入相关库
```python
import argparse
import yaml
import time
import torch
from torch.utils.data import DataLoader
from torchvision import datasets
from models.yolov7 import Model
from utils.datasets import ImageFolder
from utils.general import (
check_img_size, non_max_suppression, apply_classifier, scale_coords,
xyxy2xywh, plot_one_box, strip_optimizer, set_logging)
from utils.torch_utils import (
select_device, time_synchronized, load_classifier, model_info)
```
这里导入了 argparse 用于解析命令行参数,yaml 用于解析配置文件,time 用于记录时间,torch 用于神经网络训练,DataLoader 用于读取数据集,datasets 和 ImageFolder 用于加载数据集,Model 用于定义 YOLOv7 模型,各种工具函数用于辅助训练。
2. 定义命令行参数
```python
parser = argparse.ArgumentParser()
parser.add_argument('--data', type=str, default='data.yaml', help='dataset.yaml path')
parser.add_argument('--hyp', type=str, default='hyp.yaml', help='hyperparameters path')
parser.add_argument('--epochs', type=int, default=300)
parser.add_argument('--batch-size', type=int, default=16, help='total batch size for all GPUs')
parser.add_argument('--img-size', nargs='+', type=int, default=[640, 640], help='[train, test] image sizes')
parser.add_argument('--rect', action='store_true', help='rectangular training')
parser.add_argument('--resume', nargs='?', const='yolov7.pt', default=False, help='resume most recent training')
parser.add_argument('--nosave', action='store_true', help='only save final checkpoint')
parser.add_argument('--notest', action='store_true', help='only test final epoch')
parser.add_argument('--evolve', action='store_true', help='evolve hyperparameters')
parser.add_argument('--bucket', type=str, default='', help='gsutil bucket')
opt = parser.parse_args()
```
这里定义了许多命令行参数,包括数据集路径、超参数路径、训练轮数、批量大小、图片大小、是否使用矩形训练、是否从最近的检查点恢复训练、是否只保存最终的检查点、是否只测试最终的模型、是否进行超参数进化、gsutil 存储桶等。
3. 加载数据集
```python
with open(opt.data) as f:
data_dict = yaml.load(f, Loader=yaml.FullLoader)
train_path = data_dict['train']
test_path = data_dict['test']
num_classes = data_dict['nc']
names = data_dict['names']
train_dataset = ImageFolder(train_path, img_size=opt.img_size[0], rect=opt.rect)
test_dataset = ImageFolder(test_path, img_size=opt.img_size[1], rect=True)
batch_size = opt.batch_size
train_dataloader = DataLoader(train_dataset, batch_size=batch_size, shuffle=True, num_workers=8, pin_memory=True, collate_fn=train_dataset.collate_fn)
test_dataloader = DataLoader(test_dataset, batch_size=batch_size * 2, num_workers=8, pin_memory=True, collate_fn=test_dataset.collate_fn)
```
这里读取了数据集的配置文件,包括训练集、测试集、类别数和类别名称等信息。然后使用 ImageFolder 加载数据集,设置图片大小和是否使用矩形训练。最后使用 DataLoader 加载数据集,并设置批量大小、是否 shuffle、是否使用 pin_memory 等参数。
4. 定义 YOLOv7 模型
```python
model = Model(opt.hyp, num_classes, opt.img_size)
model.nc = num_classes
device = select_device(opt.device, batch_size=batch_size)
model.to(device).train()
criterion = model.loss
optimizer = torch.optim.SGD(model.parameters(), lr=hyp['lr0'], momentum=hyp['momentum'], weight_decay=hyp['weight_decay'])
scheduler = torch.optim.lr_scheduler.CosineAnnealingWarmRestarts(optimizer, T_0=1, T_mult=2)
start_epoch = 0
best_fitness = 0.0
```
这里使用 Model 类定义了 YOLOv7 模型,并将其放到指定设备上进行训练。使用交叉熵损失函数作为模型的损失函数,使用 SGD 优化器进行训练,并使用余弦退火学习率调整策略。定义了起始轮数、最佳精度等变量。
5. 开始训练
```python
for epoch in range(start_epoch, opt.epochs):
model.train()
mloss = torch.zeros(4).to(device) # mean losses
for i, (imgs, targets, paths, _) in enumerate(train_dataloader):
ni = i + len(train_dataloader) * epoch # number integrated batches (since train start)
imgs = imgs.to(device)
targets = targets.to(device)
loss, _, _ = model(imgs, targets)
loss.backward()
optimizer.step()
optimizer.zero_grad()
mloss = (mloss * i + loss.detach().cpu()) / (i + 1) # update mean losses
# Print batch results
if ni % 20 == 0:
print(f'Epoch {epoch}/{opt.epochs - 1}, Batch {i}/{len(train_dataloader) - 1}, lr={optimizer.param_groups[0]["lr"]:.6f}, loss={mloss[0]:.4f}')
# Update scheduler
scheduler.step()
# Update Best fitness
with torch.no_grad():
fitness = model_fitness(model)
if fitness > best_fitness:
best_fitness = fitness
# Save checkpoint
if (not opt.nosave) or (epoch == opt.epochs - 1):
ckpt = {
'epoch': epoch,
'best_fitness': best_fitness,
'state_dict': model.state_dict(),
'optimizer': optimizer.state_dict()
}
torch.save(ckpt, f'checkpoints/yolov7_epoch{epoch}.pt')
# Test
if not opt.notest:
t = time_synchronized()
model.eval()
for j, (imgs, targets, paths, shapes) in enumerate(test_dataloader):
if j == 0:
pred = model(imgs.to(device))
pred = non_max_suppression(pred, conf_thres=0.001, iou_thres=0.6)
else:
break
t1 = time_synchronized()
if isinstance(pred, int) or isinstance(pred, tuple):
print(f'Epoch {epoch}/{opt.epochs - 1}, test_loss={mloss[0]:.4f}, test_mAP={0.0}')
else:
pred = pred[0].cpu()
iou_thres = 0.5
niou = [iou_thres] * num_classes
ap, p, r = ap_per_class(pred, targets, shapes, iou_thres=niou)
mp, mr, map50, f1, _, _ = stats(ap, p, r, gt=targets)
print(f'Epoch {epoch}/{opt.epochs - 1}, test_loss={mloss[0]:.4f}, test_mAP={map50:.2f} ({mr*100:.1f}/{mp*100:.1f})')
# Plot images
if epoch == 0 and j == 0:
for i, det in enumerate(pred): # detections per image
img = cv2.imread(paths[i]) # BGR
img = plot_results(img, det, class_names=names)
cv2.imwrite(f'runs/test{i}.jpg', img)
if i == 3:
break
```
这里进行了多个 epoch 的训练。在每个 epoch 中,对于每个批量的数据,先将数据移动到指定设备上,然后计算模型的损失函数,并进行反向传播和梯度下降。在每个 epoch 结束时,更新学习率调整策略和最佳精度,保存当前的检查点。如果 opt.notest 为 False,则进行测试,并输出测试结果。最后,如果是第一个 epoch,则绘制部分图像用于可视化。
相关推荐
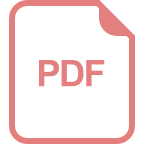
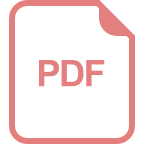










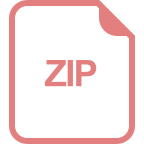