用c语言查找数组中出现次数最多的数
时间: 2023-08-08 16:14:00 浏览: 237
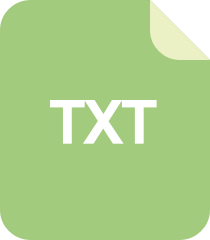
c语言数组求平均数,中位数,众数.txt
可以使用哈希表来查找出现次数最多的数。
具体步骤如下:
1. 声明一个哈希表,用来记录每个数出现的次数。
2. 遍历数组,将每个数在哈希表中对应的值加1。
3. 找到哈希表中值最大的键,即为出现次数最多的数。
以下是示例代码:
```c
#include <stdio.h>
#define SIZE 1000
int main()
{
int arr[SIZE];
int n, max_num = -1, max_count = 0;
int hash[SIZE] = {0};
printf("Enter the size of the array: ");
scanf("%d", &n);
printf("Enter the elements of the array: ");
for (int i = 0; i < n; i++) {
scanf("%d", &arr[i]);
hash[arr[i]]++;
if (hash[arr[i]] > max_count) {
max_count = hash[arr[i]];
max_num = arr[i];
}
}
printf("The number that appears the most is %d, which appears %d times.\n", max_num, max_count);
return 0;
}
```
注:此方法假设数组中所有数均为正整数且小于等于SIZE。如果不是,则需要调整SIZE的大小。
阅读全文
相关推荐
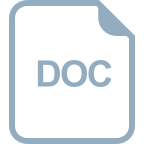
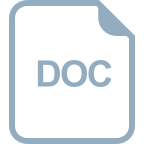
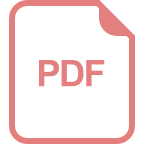



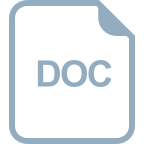
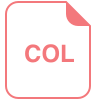









