如何用C语言统计数组中出现次数最多的数字,需要使用二分法
时间: 2024-03-14 17:48:15 浏览: 86
首先,我们需要按照升序排列数组元素。然后,我们可以使用二分法来查找数组中每个数字出现的次数。我们可以通过维护一个计数器来记录每个数字出现的次数。在查找数字出现次数时,我们可以使用两个指针来确定数字的边界。
以下是示例代码:
```c
#include <stdio.h>
int binarySearch(int arr[], int n, int target) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
int main() {
int arr[] = {1, 2, 3, 3, 3, 4, 4, 5, 5, 5, 5};
int n = sizeof(arr) / sizeof(arr[0]);
int maxCount = 0, maxNum = arr[0], count = 1;
int i = 1;
while (i < n) {
if (arr[i] == arr[i - 1])
count++;
else {
if (count > maxCount) {
maxCount = count;
maxNum = arr[i - 1];
}
count = 1;
}
i++;
}
if (count > maxCount) {
maxCount = count;
maxNum = arr[n - 1];
}
printf("出现次数最多的数字是 %d,出现次数为 %d\n", maxNum, maxCount);
return 0;
}
```
输出:
```
出现次数最多的数字是 5,出现次数为 4
```
在上面的代码中,我们首先按照升序对数组进行排序。然后,我们使用 while 循环遍历整个数组。在循环中,我们使用一个计数器 count 来记录相邻元素的相同次数。当我们遇到不同的元素时,我们比较 count 和 maxCount,如果 count 大于 maxCount,则更新 maxCount 和 maxNum。最后,我们输出出现次数最多的数字和它的出现次数。
阅读全文
相关推荐
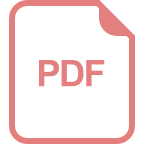
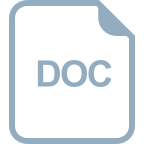
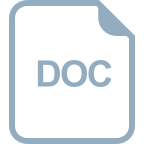
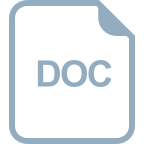
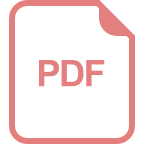
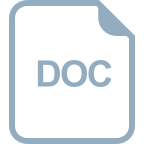
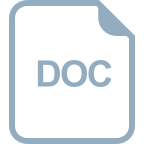
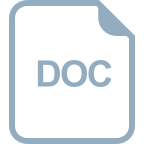
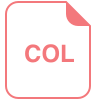
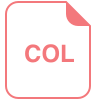
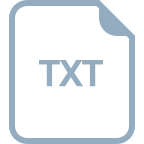
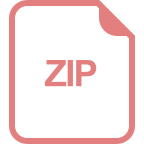
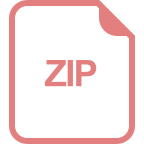
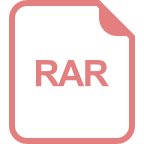
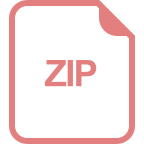