java连接sql server数据库进行查询操作
时间: 2023-08-28 19:08:12 浏览: 50
以下是Java连接SQL Server数据库进行查询操作的示例代码:
```java
import java.sql.*;
public class DatabaseQuery {
public static void main(String[] args) {
String url = "jdbc:sqlserver://localhost:1433;databaseName=mydb";
String user = "myusername";
String password = "mypassword";
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// Connect to the database
conn = DriverManager.getConnection(url, user, password);
// Create a statement object
stmt = conn.createStatement();
// Execute the SQL query
rs = stmt.executeQuery("SELECT * FROM mytable");
// Iterate through the result set and print the data
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Close the result set, statement, and connection
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例中,我们使用`DriverManager.getConnection()`方法连接到数据库。我们还创建了一个`Statement`对象并使用`executeQuery()`方法执行SQL查询。最后,我们迭代结果集并打印数据。
请注意,您需要将代码中的URL,用户名和密码替换为您自己的数据库连接信息。
相关推荐
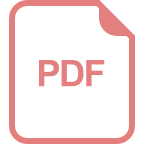
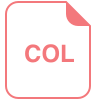
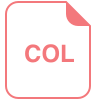
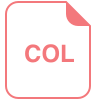
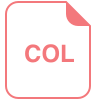
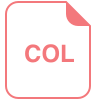









