某学生管理数据库(Mydb)中包含学生表student,表属性包括学号(id)、姓名(xm)、出生日期(csrq)、专业(zy)己声明学生Stud类,数据成员包含学生表的所有属性项,并声明读写操作。要求编写学生表的数据修改操作方法Update 注:数据库为MySQL数据库,安装在本机默认端口,库名为Mydb,root的密码为1234 Java代码
时间: 2023-06-27 08:05:46 浏览: 201
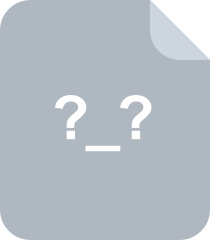
编写Student类,类中包含学生姓名,学号等私有数据成员。使用JAVA反射机制修改一个学生对象的私有数据成员

以下是Java代码示例:
```java
import java.sql.*;
public class Stud {
private String id;
private String xm;
private String csrq;
private String zy;
public Stud(String id, String xm, String csrq, String zy) {
this.id = id;
this.xm = xm;
this.csrq = csrq;
this.zy = zy;
}
public void update(String newId, String newXm, String newCsrq, String newZy) {
try {
// 加载驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 获得数据库连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/Mydb", "root", "1234");
// 编写SQL语句
String sql = "UPDATE student SET id=?, xm=?, csrq=?, zy=? WHERE id=?";
// 创建PreparedStatement对象
PreparedStatement pstmt = conn.prepareStatement(sql);
// 设置参数
pstmt.setString(1, newId);
pstmt.setString(2, newXm);
pstmt.setString(3, newCsrq);
pstmt.setString(4, newZy);
pstmt.setString(5, this.id);
// 执行SQL语句
pstmt.executeUpdate();
// 关闭资源
pstmt.close();
conn.close();
// 更新对象的属性
this.id = newId;
this.xm = newXm;
this.csrq = newCsrq;
this.zy = newZy;
System.out.println("Update success!");
} catch (Exception e) {
e.printStackTrace();
}
}
// 省略读写操作的方法
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
Stud stud = new Stud("001", "张三", "1999-01-01", "计算机科学");
stud.update("002", "李四", "2000-02-02", "软件工程");
System.out.println(stud.getId() + " " + stud.getXm() + " " + stud.getCsrq() + " " + stud.getZy());
}
}
```
上述代码中,通过JDBC连接MySQL数据库,执行UPDATE语句来修改学生表中的记录,并更新Stud对象的属性。注意,在update方法中,this.id是原始学生的id,而pstmt.setString(5, this.id)是设置WHERE子句的条件。最后,执行完UPDATE语句后,需要关闭PreparedStatement和Connection对象。
阅读全文
相关推荐
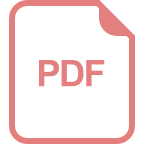
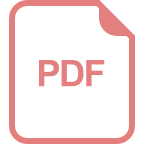





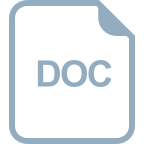









